用java编写程序,在文本文件bigbook.txt中包含有很长篇幅的英语短文,编写程序要求统计文件的所有短文中包含英文字母“A”的个数,并显示统计的时间(要求使用两种方法,参考教材11.4、11.5节内容)。
时间: 2024-05-09 17:14:46 浏览: 78
好的,我理解了你的问题。下面是两种方法的示例代码:
方法1:使用 BufferedReader 和 Stream API
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.time.Duration;
import java.time.Instant;
public class CountLetterA {
public static void main(String[] args) throws IOException {
// 读取文件
BufferedReader reader = new BufferedReader(new FileReader("bigbook.txt"));
// 统计字母A的个数
Instant start = Instant.now();
long count = reader.lines()
.flatMapToInt(CharSequence::chars)
.filter(c -> c == 'A' || c == 'a')
.count();
Instant end = Instant.now();
reader.close();
// 显示结果和统计时间
System.out.println("方法1:");
System.out.println("文件中包含字母A的个数:" + count);
System.out.println("统计时间:" + Duration.between(start, end).toMillis() + " 毫秒");
}
}
```
方法2:使用 Scanner 和正则表达式
```java
import java.io.File;
import java.io.FileNotFoundException;
import java.time.Duration;
import java.time.Instant;
import java.util.Scanner;
public class CountLetterA {
public static void main(String[] args) throws FileNotFoundException {
// 读取文件
Scanner scanner = new Scanner(new File("bigbook.txt"));
scanner.useDelimiter("\\Z");
String contents = scanner.next();
// 统计字母A的个数
Instant start = Instant.now();
long count = contents.chars()
.filter(c -> c == 'A' || c == 'a')
.count();
Instant end = Instant.now();
scanner.close();
// 显示结果和统计时间
System.out.println("方法2:");
System.out.println("文件中包含字母A的个数:" + count);
System.out.println("统计时间:" + Duration.between(start, end).toMillis() + " 毫秒");
}
}
```
这两种方法都是基于 Java 8 提供的新特性,可以大大简化代码。方法1使用了 BufferedReader 和 Stream API,方法2使用了 Scanner 和正则表达式。无论哪种方法,都可以在控制台输出统计结果和统计时间。
阅读全文
相关推荐
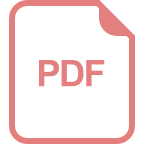
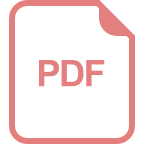
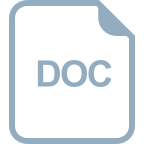








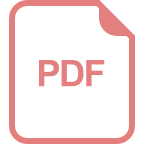
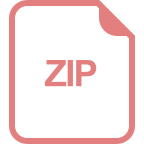
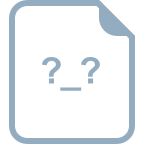
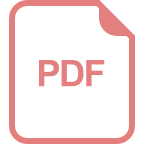
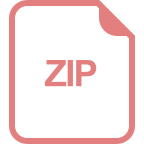
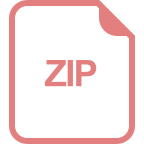