Ruoyi导出多个excel为zip
时间: 2024-05-03 11:21:53 浏览: 62
您可以使用Java的ZipOutputStream类来实现将多个Excel文件打包成一个ZIP文件。以下是一个基本示例:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class ExcelZipper {
public static void main(String[] args) {
// Source directory containing the Excel files
String sourceDir = "/path/to/excel/files/";
// Destination directory for the ZIP file
String destDir = "/path/to/zip/output/";
// Name of the ZIP file
String zipName = "excel_files.zip";
try {
// Create a FileOutputStream for the ZIP file
FileOutputStream fos = new FileOutputStream(destDir + zipName);
// Create a ZipOutputStream for the FileOutputStream
ZipOutputStream zos = new ZipOutputStream(fos);
// Get a list of all the Excel files in the source directory
File[] excelFiles = new File(sourceDir).listFiles((dir, name) -> name.endsWith(".xlsx") || name.endsWith(".xls"));
// Loop through each Excel file and add it to the ZIP file
for (File file : excelFiles) {
// Create a new ZipEntry for the file
ZipEntry zipEntry = new ZipEntry(file.getName());
// Add the ZipEntry to the ZipOutputStream
zos.putNextEntry(zipEntry);
// Create a FileInputStream for the Excel file
FileInputStream fis = new FileInputStream(file);
// Read the Excel file and write it to the ZipOutputStream
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) > 0) {
zos.write(buffer, 0, length);
}
// Close the FileInputStream
fis.close();
// Close the ZipEntry
zos.closeEntry();
}
// Close the ZipOutputStream
zos.close();
System.out.println("Excel files have been zipped successfully!");
} catch (Exception ex) {
ex.printStackTrace();
System.out.println("Error zipping Excel files: " + ex.getMessage());
}
}
}
```
您需要将“/path/to/excel/files/”替换为包含Excel文件的实际源目录,“/path/to/zip/output/”替换为ZIP文件的实际目标目录,并将“excel_files.zip”替换为所需的ZIP文件名称。
此代码将创建一个ZipOutputStream并将Excel文件添加到其中。ZipOutputStream将自动将Excel文件压缩为ZIP文件。一旦所有文件都添加到ZipOutputStream中,就可以关闭它并将所有Excel文件打包到单个ZIP文件中。
请注意,此代码仅适用于XLSX和XLS格式的Excel文件。如果您需要支持其他格式,请相应地修改文件过滤器。
希望这可以帮助您实现所需的功能。
阅读全文
相关推荐
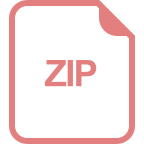
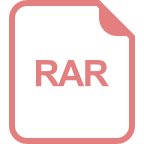















