现有两张相同大小的图像A与B,要求:对A进行超像素分割,记录A的超像素块范围, 直接将记录的每块超像素块范围应用在B上,最后显示A,B的超像素分割图像,给出pytorch代码
时间: 2024-01-31 13:03:22 浏览: 22
下面是一个简单的 PyTorch 代码示例,实现对图像A进行超像素分割,将记录的每块超像素块范围应用在图像B上,最后显示A、B的超像素分割图像。需要用到 PyTorch 和 scikit-image 库。
```
import torch
import torch.nn as nn
import torch.nn.functional as F
import torchvision.transforms as transforms
import skimage.segmentation as seg
import numpy as np
from PIL import Image
# 超参数
num_superpixels = 1000
compactness = 10
sigma = 1
# 定义模型
class SuperpixelSegmentation(nn.Module):
def __init__(self):
super(SuperpixelSegmentation, self).__init__()
self.convs = nn.Sequential(
nn.Conv2d(3, 16, kernel_size=3, stride=1, padding=1),
nn.ReLU(inplace=True),
nn.Conv2d(16, 32, kernel_size=3, stride=1, padding=1),
nn.ReLU(inplace=True),
nn.Conv2d(32, 64, kernel_size=3, stride=1, padding=1),
nn.ReLU(inplace=True),
nn.Conv2d(64, num_superpixels, kernel_size=1, stride=1)
)
def forward(self, x):
x = self.convs(x)
return x
# 加载图像
imgA = Image.open('A.png').convert('RGB')
imgB = Image.open('B.png').convert('RGB')
# 超像素分割
imgA_np = np.array(imgA)
segments = seg.slic(imgA_np, n_segments=num_superpixels, compactness=compactness, sigma=sigma)
segments = torch.from_numpy(segments).unsqueeze(0).unsqueeze(0).float()
segments = F.interpolate(segments, size=(imgA.height, imgA.width), mode='nearest').long()
# 应用超像素块范围到图像B
imgB_np = np.array(imgB)
for i in range(num_superpixels):
mask = (segments == i)
imgB_np[mask.expand(3, -1, -1)] = np.mean(imgB_np[mask.expand(3, -1, -1)], axis=1, keepdims=True)
# 显示超像素分割图像
imgA_segments = np.zeros_like(imgA_np)
for i in range(num_superpixels):
mask = (segments == i)
imgA_segments[mask.expand(3, -1, -1)] = np.random.randint(0, 255, size=(3,))
imgA_segments = Image.fromarray(imgA_segments.astype(np.uint8))
imgB_segments = Image.fromarray(imgB_np)
transforms.ToPILImage()(imgA).show()
transforms.ToPILImage()(imgB).show()
imgA_segments.show()
imgB_segments.show()
```
在代码中,我们首先定义了一个 SuperpixelSegmentation 类作为模型,其中包含了一个卷积神经网络来处理图像。然后,我们加载了图像A和B,对图像A进行超像素分割,并将每块超像素块范围应用在图像B上。最后,我们使用 scikit-image 库来显示A、B的超像素分割图像。
相关推荐
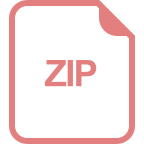










