python实现k折交叉验证
时间: 2023-10-02 15:03:44 浏览: 108
K折交叉验证是一种常用的模型评估方法,可以在有限的数据集上尽可能地利用数据来训练和测试模型。在Python中,可以使用scikit-learn库来实现K折交叉验证。以下是一个基本的代码示例:
```python
from sklearn.model_selection import KFold
from sklearn.linear_model import LinearRegression
import numpy as np
# 准备数据
X = np.array([[1, 2], [3, 4], [5, 6], [7, 8]])
y = np.array([1, 2, 3, 4])
# 定义K折交叉验证
kfold = KFold(n_splits=2, shuffle=True, random_state=0)
# 定义模型
model = LinearRegression()
# 进行K折交叉验证
for train_index, test_index in kfold.split(X):
X_train, X_test = X[train_index], X[test_index]
y_train, y_test = y[train_index], y[test_index]
model.fit(X_train, y_train)
print("Train Score:", model.score(X_train, y_train))
print("Test Score:", model.score(X_test, y_test))
```
在上面的示例中,我们首先准备了一个包含4个样本和2个特征的数据集,并将目标变量设置为y。然后,我们使用scikit-learn库中的KFold类来定义一个2折交叉验证,并将数据集拆分为训练集和测试集。
在每次迭代中,我们从KFold对象中获取训练集和测试集的索引,并将它们用于获取相应的训练数据和测试数据。然后,我们使用训练数据拟合模型,并分别计算训练集得分和测试集得分。
最后,我们可以将训练集得分和测试集得分输出到控制台上。这样我们就可以了解模型在K折交叉验证的每个折叠中的性能表现。
阅读全文
相关推荐
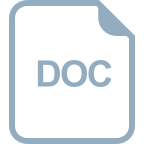
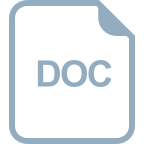
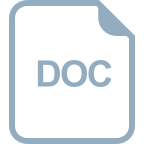
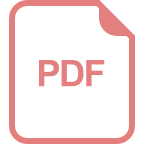
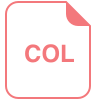
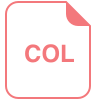


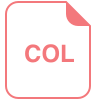








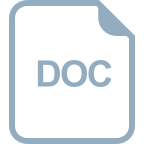
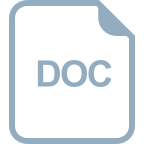
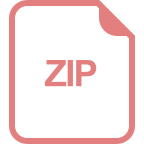