Set<ScheduledTask> scheduledTasks = scheduledTaskRegistrar.getScheduledTasks(); 停止单个任务
时间: 2023-05-27 21:02:08 浏览: 59
如果要停止单个任务,需要先获取所有已经安排的任务,然后找到要停止的任务,并调用其取消方法即可。
下面是一个示例代码:
```
Set<ScheduledTask> scheduledTasks = scheduledTaskRegistrar.getScheduledTasks();
for (ScheduledTask task : scheduledTasks) {
if (task.getRunnable() instanceof MyScheduledTask) { // 假设要停止的任务类型是 MyScheduledTask
MyScheduledTask myTask = (MyScheduledTask) task.getRunnable();
if (myTask.getId().equals("task001")) { // 假设要停止的任务 ID 是 task001
boolean result = task.cancel(); // 停止任务并返回是否成功
if (result) {
System.out.println("任务已停止。");
} else {
System.out.println("停止任务失败。");
}
break; // 找到并停止了任务,退出循环
}
}
}
```
这段代码首先获取所有已经安排的任务,遍历每个任务并检查类型和 ID 是否符合要求。如果找到了要停止的任务,就调用其取消方法,停止任务并输出结果。需要注意的是,必须在找到并停止了任务后,退出循环,否则还可能停止其他符合条件的任务。
相关推荐
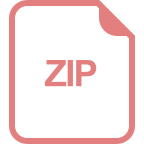
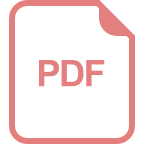














