在vue中怎么去重
时间: 2023-07-16 16:16:56 浏览: 116
在 Vue 中进行数组去重,可以使用 JavaScript 的 Set 对象。Set 对象是一种集合,其中的元素不会重复,可以通过 Array.from() 方法将 Set 转换为数组。
下面是一个示例代码,使用 Set 对象对数组进行去重:
```
<template>
<div>
<ul>
<li v-for="item in uniqueList">{{ item }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
list: [1, 2, 3, 4, 4, 5, 5, 6]
};
},
computed: {
uniqueList() {
return Array.from(new Set(this.list));
}
}
};
</script>
```
上面的代码中,我们使用 Set 对象将 this.list 去重,并使用 computed 计算属性将结果返回。在模板中,我们通过 v-for 指令遍历 uniqueList 数组,从而显示去重后的数据列表。
相关问题
vue3去重
在 Vue 3 中,可以使用 `reactive` 函数创建响应式对象,再使用 `watch` 函数监听数组的变化,在回调函数中使用 `Set` 数据结构进行去重。示例代码如下:
```
import { reactive, watch } from 'vue';
const state = reactive({
arr: [1, 2, 2, 3, 4, 4, 5],
});
watch(
() => state.arr,
(newVal, oldVal) => {
state.arr = Array.from(new Set(newVal));
}
);
console.log(state.arr); // [1, 2, 3, 4, 5]
```
在上面的代码中,首先使用 `reactive` 函数创建 `state` 对象,其中包含一个数组 `arr`。然后使用 `watch` 函数监听 `arr` 数组的变化,当 `arr` 数组发生变化时,回调函数会将 `arr` 数组转换为 `Set` 数据结构并去重,最后将去重后的数组赋值给 `state.arr`。
需要注意的是,在 Vue 3 中,`watch` 函数默认是“深度监听”的,也就是说,当数组中的某个元素发生变化时,也会触发回调函数。如果不需要监听数组中元素的变化,可以在 `watch` 函数的第三个参数中传入 `{ deep: false }`,如下所示:
```
watch(
() => state.arr,
(newVal, oldVal) => {
state.arr = Array.from(new Set(newVal));
},
{ deep: false }
);
```
这样就只会监听数组的变化,而不会监听数组中元素的变化。
vue list去重
在 Vue 中对列表进行去重有多种方法。以下是几种常见的方法:
1. 使用 JavaScript 的 Set 数据结构:将列表转换为 Set,然后再转换回数组。
```javascript
const uniqueList = Array.from(new Set(yourList));
```
2. 使用 filter 方法:遍历列表,只保留第一次出现的元素。
```javascript
const uniqueList = yourList.filter((item, index) => yourList.indexOf(item) === index);
```
3. 使用 reduce 方法:遍历列表,将第一次出现的元素添加到结果数组中。
```javascript
const uniqueList = yourList.reduce((acc, curr) => {
if (!acc.includes(curr)) {
acc.push(curr);
}
return acc;
}, []);
```
请注意,这些方法都会创建一个新的去重后的数组。如果你希望在原始列表上进行修改,请相应地调整代码。
阅读全文
相关推荐















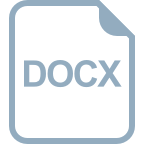