用java把三个成绩的总成绩排序_用java编一个学生成绩排序成绩 有学生的姓名 学号 三门课的成绩 然后对总分排序...
时间: 2024-03-17 14:45:51 浏览: 60
好的,这个问题很简单。以下是一个Java程序,可以读取学生姓名、学号和三门课的成绩,并且按照总分进行排序:
```java
import java.util.*;
class Student implements Comparable<Student> {
String name;
int id;
int score1, score2, score3;
int total;
public Student(String name, int id, int score1, int score2, int score3) {
this.name = name;
this.id = id;
this.score1 = score1;
this.score2 = score2;
this.score3 = score3;
this.total = score1 + score2 + score3;
}
public int compareTo(Student other) {
return other.total - this.total;
}
public String toString() {
return String.format("%s (%d): %d", name, id, total);
}
}
public class SortStudents {
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
students.add(new Student("Alice", 1, 80, 70, 90));
students.add(new Student("Bob", 2, 70, 60, 80));
students.add(new Student("Charlie", 3, 90, 80, 70));
students.add(new Student("David", 4, 60, 80, 90));
Collections.sort(students);
for (Student s : students) {
System.out.println(s);
}
}
}
```
注意,这个程序中的 `Student` 类实现了 `Comparable` 接口,因此我们可以使用 `Collections.sort()` 方法对学生列表进行排序。排序的依据是 `total` 属性,即三门课的总分。
阅读全文
相关推荐


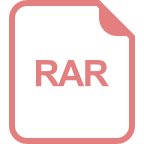


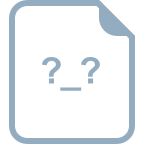
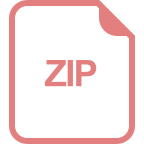
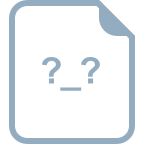