c++中如何定义string类型的数组
时间: 2024-05-04 07:18:13 浏览: 144
在 C 语言中,可以使用字符类型的数组来表示字符串。可以定义一个字符类型的数组来表示一个字符串数组,例如:
```c
char str[100];
```
这个定义创建了一个字符类型的数组,其大小为 100 个字符,可以存储一个最大长度为 99 的字符串,因为最后一个位置必须留给字符串结束符 '\0'。
相关问题
c++中给string类型数组开空间
可以使用如下代码给string类型的数组开空间:
```c
#include <string.h>
#include <stdlib.h>
#include <stdio.h>
int main() {
const int size = 10;
char **string_array = malloc(size * sizeof(char *));
if (string_array == NULL) {
printf("Failed to allocate memory.\n");
return 1;
}
for (int i = 0; i < size; ++i) {
string_array[i] = malloc(256 * sizeof(char));
if (string_array[i] == NULL) {
printf("Failed to allocate memory.\n");
return 1;
}
}
printf("Successfully allocated memory for string array.\n");
// TODO: do something with string array
// Free memory
for (int i = 0; i < size; ++i) {
free(string_array[i]);
}
free(string_array);
return 0;
}
```
c++中string类型的数组怎么输入
您可以使用 `std::getline()` 函数将文本输入到 `std::string` 数组中。例如,以下代码将输入3个字符串并将它们存储在 `std::string` 数组中:
```c++
#include <iostream>
#include <string>
int main() {
const int SIZE = 3;
std::string strArr[SIZE];
for (int i = 0; i < SIZE; i++) {
std::cout << "Enter a string: ";
std::getline(std::cin, strArr[i]);
}
std::cout << "The strings you entered are: " << std::endl;
for (int i = 0; i < SIZE; i++) {
std::cout << strArr[i] << std::endl;
}
return 0;
}
```
在上面的代码中,我们首先定义了一个大小为3的 `std::string` 类型的数组 `strArr`。然后使用 `std::getline()` 函数将用户输入的字符串存储到数组中。最后,我们遍历数组并将输入的字符串输出到屏幕上。
阅读全文
相关推荐
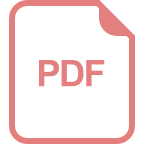
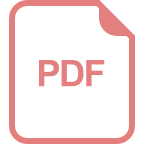
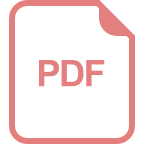













