android实现一个简易电话簿、打电话、发短信代码和布局文件
时间: 2023-12-18 11:04:25 浏览: 170
以下是一个简易电话簿的实现,包括打电话和发送短信功能。需要注意的是,需要在 AndroidManifest.xml 文件中添加对应的权限。
布局文件 activity_main.xml:
```xml
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<ListView
android:id="@+id/contact_list"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:padding="16dp">
<EditText
android:id="@+id/search_text"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:hint="Search contacts" />
<Button
android:id="@+id/search_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Search" />
</LinearLayout>
</LinearLayout>
```
联系人列表项布局文件 contact_item.xml:
```xml
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/name_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp"
android:textStyle="bold" />
<TextView
android:id="@+id/phone_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="16sp" />
<TextView
android:id="@+id/email_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="16sp" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/call_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Call" />
<Button
android:id="@+id/sms_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="SMS" />
</LinearLayout>
</LinearLayout>
```
Java 代码 MainActivity.java:
```java
public class MainActivity extends AppCompatActivity {
private List<Contact> contactList;
private ContactAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 初始化联系人列表
contactList = new ArrayList<>();
adapter = new ContactAdapter(this, contactList);
ListView contactListView = findViewById(R.id.contact_list);
contactListView.setAdapter(adapter);
// 添加联系人
Contact contact1 = new Contact("John Smith", "1234567890", "john.smith@example.com");
Contact contact2 = new Contact("Jane Doe", "0987654321", "jane.doe@example.com");
Contact contact3 = new Contact("Bob Johnson", "5555555555", "bob.johnson@example.com");
contactList.add(contact1);
contactList.add(contact2);
contactList.add(contact3);
// 设置联系人列表项点击事件
contactListView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Contact contact = contactList.get(position);
String phone = contact.getPhone();
String email = contact.getEmail();
// 打电话
Intent callIntent = new Intent(Intent.ACTION_CALL, Uri.parse("tel:" + phone));
startActivity(callIntent);
// 发短信
Intent smsIntent = new Intent(Intent.ACTION_SENDTO, Uri.parse("smsto:" + phone));
smsIntent.putExtra("sms_body", "Hello, " + contact.getName() + "!");
startActivity(smsIntent);
}
});
// 设置搜索按钮点击事件
Button searchButton = findViewById(R.id.search_button);
searchButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
EditText searchText = findViewById(R.id.search_text);
String query = searchText.getText().toString().toLowerCase();
List<Contact> filteredList = new ArrayList<>();
for (Contact contact : contactList) {
if (contact.getName().toLowerCase().contains(query)
|| contact.getPhone().contains(query)
|| contact.getEmail().toLowerCase().contains(query)) {
filteredList.add(contact);
}
}
adapter.clear();
adapter.addAll(filteredList);
adapter.notifyDataSetChanged();
}
});
}
}
```
联系人数据模型类 Contact.java:
```java
public class Contact {
private String name;
private String phone;
private String email;
public Contact(String name, String phone, String email) {
this.name = name;
this.phone = phone;
this.email = email;
}
public String getName() {
return name;
}
public String getPhone() {
return phone;
}
public String getEmail() {
return email;
}
}
```
联系人列表适配器类 ContactAdapter.java:
```java
public class ContactAdapter extends ArrayAdapter<Contact> {
public ContactAdapter(Context context, List<Contact> contacts) {
super(context, 0, contacts);
}
@NonNull
@Override
public View getView(int position, @Nullable View convertView, @NonNull ViewGroup parent) {
if (convertView == null) {
convertView = LayoutInflater.from(getContext()).inflate(R.layout.contact_item, parent, false);
}
Contact contact = getItem(position);
TextView nameText = convertView.findViewById(R.id.name_text);
nameText.setText(contact.getName());
TextView phoneText = convertView.findViewById(R.id.phone_text);
phoneText.setText(contact.getPhone());
TextView emailText = convertView.findViewById(R.id.email_text);
emailText.setText(contact.getEmail());
Button callButton = convertView.findViewById(R.id.call_button);
callButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String phone = contact.getPhone();
Intent callIntent = new Intent(Intent.ACTION_CALL, Uri.parse("tel:" + phone));
getContext().startActivity(callIntent);
}
});
Button smsButton = convertView.findViewById(R.id.sms_button);
smsButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String phone = contact.getPhone();
Intent smsIntent = new Intent(Intent.ACTION_SENDTO, Uri.parse("smsto:" + phone));
smsIntent.putExtra("sms_body", "Hello, " + contact.getName() + "!");
getContext().startActivity(smsIntent);
}
});
return convertView;
}
}
```
阅读全文
相关推荐
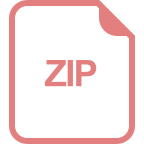
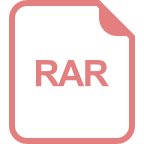
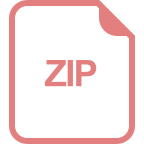















