python的opencv画虚线
时间: 2023-06-02 16:03:36 浏览: 215
你好,关于Python中OpenCV如何画虚线,请参考以下代码:
```
import cv2
import numpy as np
# 创建黑色的图像
img = np.zeros((512,512,3), np.uint8)
# 画一条虚线
cv2.line(img,(0,0),(511,511),(255,0,0),5,cv2.LINE_AA)
# 显示图像
cv2.imshow("Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`cv2.line()`函数用于画线,参数依次为:
- 图像
- 起点坐标
- 终点坐标
- 颜色(BGR 格式)
- 线宽
- 线条类型(`cv2.LINE_AA` 表示抗锯齿)
如需绘制虚线,只需要将线条类型设置为`cv2.LINE_DASH`即可。如有疑问,请随时询问。
相关问题
python画虚线的矩形框
你可以使用Python中的OpenCV库来画虚线的矩形框。下面是一个示例代码:
```python
import cv2
# 读取图像
img = cv2.imread('image.jpg')
# 定义矩形框的起点和终点坐标
start_point = (50, 50)
end_point = (200, 200)
# 定义矩形框的颜色和线宽
color = (255, 0, 0)
thickness = 2
# 画虚线的矩形框
cv2.rectangle(img, start_point, end_point, color, thickness, cv2.LINE_AA)
# 显示图像
cv2.imshow("Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上面的代码中,我们使用`cv2.rectangle()`函数来画矩形框,并将线型设置为`cv2.LINE_AA`,即抗锯齿线型,从而实现画虚线的效果。
用opencv写一个画虚线的函数
以下是用 OpenCV 在图片上画虚线的函数:
```python
import numpy as np
import cv2
def draw_dashed_line(img, pt1, pt2, color=(0, 0, 255), thickness=2, dash_length=10, gap_length=5):
"""在图片上画虚线"""
dist = np.sqrt((pt2[0] - pt1[0])**2 + (pt2[1] - pt1[1])**2)
pts = []
for i in np.arange(0, dist, dash_length + gap_length):
r = i / dist
x = int((1 - r) * pt1[0] + r * pt2[0])
y = int((1 - r) * pt1[1] + r * pt2[1])
pts.append((x, y))
if len(pts) % 2 == 1:
pts = pts[:-1]
for i in range(0, len(pts), 2):
cv2.line(img, pts[i], pts[i+1], color, thickness)
# 测试
img = np.zeros((512, 512, 3), np.uint8)
draw_dashed_line(img, (50, 50), (200, 200), (0, 255, 0), 3, 10, 5)
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这个函数的参数包括:
- `img`: 要画虚线的图片,必须是一个 OpenCV 的 `numpy.ndarray` 对象。
- `pt1` 和 `pt2`: 虚线的起点和终点,坐标可以是整数或浮点数。
- `color`: 虚线的颜色,可以是一个三个元素的元组或列表,分别对应蓝色、绿色和红色的值,范围是 0~255。
- `thickness`: 虚线的粗细,整数类型。
- `dash_length` 和 `gap_length`: 虚线和空白部分的长度,两个参数都是整数类型。
在上面的例子中,我们在一个黑色的图片上画了一条起点为 (50, 50)、终点为 (200, 200)、颜色为绿色、粗细为 3、虚线长度为 10、空白长度为 5 的虚线。你可以根据需要修改参数来画出不同的虚线。
阅读全文
相关推荐
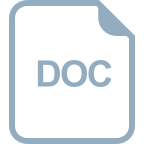
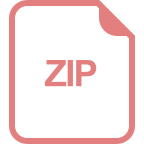
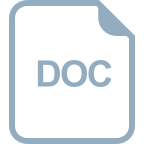
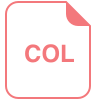


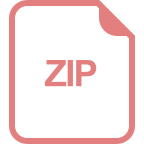
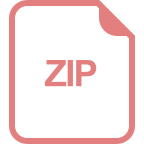
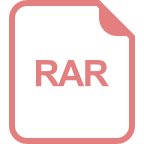
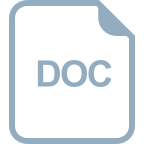
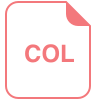
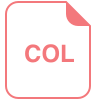
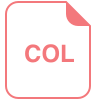
