用jsp+struts2框架代码实现以下功能:导出千万级数据量,要求每50000笔数据导入到excel表格直到导完为止,再讲得到的多个excel表格压缩成zip包,最后导出为一个压缩包
时间: 2023-08-09 15:04:55 浏览: 181
首先,我们需要准备好以下几个工具:
1. Apache POI:用于操作 Excel 文件。
2. ZipOutputStream:用于将多个文件压缩成一个 Zip 包。
接下来,我们先来看看如何将数据导出到 Excel 文件中。
1. 在 JSP 页面中添加一个导出按钮,点击该按钮后触发一个 action。
2. 在 Struts2 中创建一个 action,该 action 会调用一个 service 方法,该方法用于从数据库中查询数据,并将数据写入 Excel 文件中。
3. 在 service 方法中,我们需要分批次查询数据,每次查询 50000 条数据,然后将这些数据写入一个 Excel 文件中。
```java
// 创建 Excel 文件
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sheet1");
// 写入数据
for (int i = 0; i < dataList.size(); i++) {
Row row = sheet.createRow(i);
// 写入数据
...
}
// 保存 Excel 文件
FileOutputStream out = new FileOutputStream(filePath);
workbook.write(out);
out.close();
```
4. 当每个 Excel 文件都写好后,我们需要将它们压缩成一个 Zip 包。可以使用 ZipOutputStream 类来完成这个任务。
```java
ZipOutputStream zos = new ZipOutputStream(new FileOutputStream(zipPath));
for (String excelPath : excelPaths) {
File excelFile = new File(excelPath);
FileInputStream fis = new FileInputStream(excelFile);
byte[] buffer = new byte[1024];
zos.putNextEntry(new ZipEntry(excelFile.getName()));
int len;
while ((len = fis.read(buffer)) > 0) {
zos.write(buffer, 0, len);
}
fis.close();
zos.closeEntry();
}
zos.close();
```
5. 最后,将生成的 Zip 包返回给用户。
完整代码如下:
JSP 页面:
```html
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="s" uri="/struts-tags"%>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>导出数据</title>
</head>
<body>
<s:form action="exportData">
<s:submit value="导出数据" />
</s:form>
</body>
</html>
```
Struts2 action:
```java
public class ExportDataAction extends ActionSupport {
private static final long serialVersionUID = 1L;
public String execute() throws Exception {
// 查询数据
List<Data> dataList = getDataFromDB();
// 将数据写入 Excel 文件中
int batchSize = 50000;
int fileCount = 1;
List<String> excelPaths = new ArrayList<>();
for (int i = 0; i < dataList.size(); i += batchSize) {
int endIndex = Math.min(i + batchSize, dataList.size());
List<Data> batchList = dataList.subList(i, endIndex);
String excelPath = "data_" + fileCount + ".xlsx";
writeToExcel(batchList, excelPath);
excelPaths.add(excelPath);
fileCount++;
}
// 将多个 Excel 文件压缩成一个 Zip 包
String zipPath = "data.zip";
writeToZip(excelPaths, zipPath);
// 下载 Zip 包
downloadFile(zipPath);
return null;
}
private List<Data> getDataFromDB() {
// 从数据库中查询数据
...
}
private void writeToExcel(List<Data> dataList, String filePath) throws IOException {
// 创建 Excel 文件
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sheet1");
// 写入数据
for (int i = 0; i < dataList.size(); i++) {
Row row = sheet.createRow(i);
// 写入数据
...
}
// 保存 Excel 文件
FileOutputStream out = new FileOutputStream(filePath);
workbook.write(out);
out.close();
}
private void writeToZip(List<String> excelPaths, String zipPath) throws IOException {
ZipOutputStream zos = new ZipOutputStream(new FileOutputStream(zipPath));
for (String excelPath : excelPaths) {
File excelFile = new File(excelPath);
FileInputStream fis = new FileInputStream(excelFile);
byte[] buffer = new byte[1024];
zos.putNextEntry(new ZipEntry(excelFile.getName()));
int len;
while ((len = fis.read(buffer)) > 0) {
zos.write(buffer, 0, len);
}
fis.close();
zos.closeEntry();
}
zos.close();
}
private void downloadFile(String filePath) throws IOException {
HttpServletResponse response = ServletActionContext.getResponse();
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment;filename=" + URLEncoder.encode(filePath, "UTF-8"));
InputStream in = new FileInputStream(filePath);
OutputStream out = response.getOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = in.read(buffer)) > 0) {
out.write(buffer, 0, len);
}
in.close();
out.close();
}
}
```
阅读全文
相关推荐
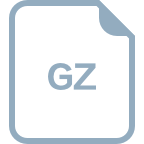















