wmi 启动远程exe
时间: 2023-08-02 15:05:11 浏览: 56
可以使用 WMI (Windows Management Instrumentation) 来启动远程的 EXE 文件。以下是一个示例 PowerShell 脚本:
```powershell
$computerName = "RemoteComputerName"
$processName = "C:\Path\To\Your\Executable.exe"
$mc = [System.Management.ManagementClass]"\\$computerName\root\cimv2:Win32_Process"
$result = $mc.create($processName)
```
将 `RemoteComputerName` 替换为要启动 EXE 文件的远程计算机的名称,将 `C:\Path\To\Your\Executable.exe` 替换为要启动的 EXE 文件的路径。
请注意,要运行此脚本,您需要在远程计算机上具有管理员权限。
相关问题
wmitools.exe
wmitools.exe是一个用于管理和操作Windows管理体系结构(WMI)的工具。WMI是微软开发的一种用于管理Windows操作系统及其相关组件的技术,可以通过WMI来查询系统状态、监视性能、进行系统配置和管理等各种操作。
通过运行wmitools.exe可以打开一个命令行界面,然后可以输入各种命令来实现对WMI的操作。例如,可以使用wmitools.exe来查询系统的硬件信息,如处理器、内存、硬盘等。也可以使用该工具来查询系统的软件信息,如安装的应用程序、服务和驱动程序等。此外,还可以使用wmitools.exe来创建和执行WMI查询语言(WQL)语句,从而实现对系统的更深层次的查询和控制。
wmitools.exe提供了许多功能强大的命令,可以用于对WMI命名空间、类和实例进行操作。它还支持WMI事件订阅和作业调度,可以帮助用户实现自动化任务和事件监控。
总之,wmitools.exe是一个非常有用的工具,它可以帮助用户更方便地管理和操作Windows管理体系结构。无论是进行系统的日常管理,还是进行更深入的系统调试和故障排除,都可以通过wmitools.exe来实现。它的使用可以提高系统管理的效率和准确性,使用户能更好地了解和控制系统的各个方面。
wmiprvse.exe wmi provider host
wmiprvse.exe是Windows操作系统中的一个进程,在任务管理器中可以看到它的运行。它是WMI(Windows Management Instrumentation)提供程序主机的缩写,是Windows系统的一个重要组件。
WMI是一种微软公司提供的管理和监控操作系统、应用程序和网络的技术。它通过提供一种统一的接口,使得管理员可以在远程或本地管理和监控Windows操作系统上的各种资源,如进程、服务、硬件等。
wmiprvse.exe是WMI提供程序主机的主要进程。它负责加载和运行所有的WMI提供程序,这些提供程序可以收集系统信息、执行系统管理任务,以及提供其他的管理功能。由于这些提供程序可以在后台运行,所以wmiprvse.exe可能会消耗一定的系统资源,如CPU和内存。
通常情况下,wmiprvse.exe是一个合法和安全的进程,但有时候会出现该进程异常占用系统资源的情况,导致系统变得缓慢或不稳定。这可能是由于一些WMI提供程序出现问题或冲突导致的。在这种情况下,可以尝试通过重启计算机或重新注册WMI服务来解决问题。
总之,wmiprvse.exe是Windows系统中负责运行WMI提供程序的进程,它提供了一种统一的管理和监控接口,使管理员能够方便地管理和监控系统资源。
相关推荐
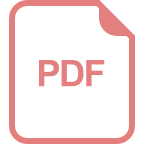
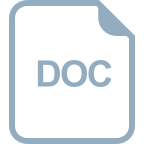
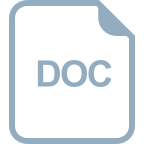












