使用socket封装一个java可执行读写redis命令并获取返回值的工具类
时间: 2023-11-27 12:51:49 浏览: 114
以下是一个使用Socket封装的Java Redis工具类,可以用于执行Redis命令并获取返回值。
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.Socket;
public class RedisClient {
private String host;
private int port;
private Socket socket;
private BufferedReader reader;
private OutputStream writer;
public RedisClient(String host, int port) {
this.host = host;
this.port = port;
}
public void connect() throws IOException {
socket = new Socket(host, port);
reader = new BufferedReader(new InputStreamReader(socket.getInputStream()));
writer = socket.getOutputStream();
}
public String sendCommand(String command) throws IOException {
// 发送命令
writer.write(command.getBytes());
writer.write("\r\n".getBytes());
writer.flush();
// 读取返回值
StringBuilder response = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
if (line.startsWith("+") || line.startsWith("-") || line.startsWith(":")) {
// 状态回复、错误回复、整数回复
response.append(line.substring(1)).append("\n");
break;
} else if (line.startsWith("$")) {
// 批量回复
int length = Integer.parseInt(line.substring(1));
if (length == -1) {
response.append("null\n");
} else {
char[] buffer = new char[length];
reader.read(buffer);
reader.read(); // 读取分隔符
response.append(new String(buffer)).append("\n");
}
break;
} else if (line.startsWith("*")) {
// 多条批量回复
int count = Integer.parseInt(line.substring(1));
for (int i = 0; i < count; i++) {
response.append(sendCommand("")); // 递归调用,读取每个元素
}
break;
}
}
return response.toString();
}
public void close() throws IOException {
socket.close();
}
}
```
使用方法如下:
```java
RedisClient client = new RedisClient("127.0.0.1", 6379);
client.connect();
String result = client.sendCommand("PING");
System.out.println(result);
client.close();
```
上述代码会连接到本地的Redis服务器,并执行PING命令,然后打印返回值。你可以根据需要修改代码以适应其他Redis命令。
阅读全文
相关推荐









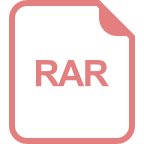

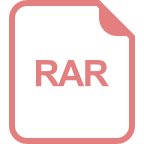






