使用C++编译一个停车场管理系统,其中有汽车信息,普通用户,管理用户三个模块,普通用户只能查看车辆信息,管理用户能添加修改删除车辆信息
时间: 2023-11-11 14:03:19 浏览: 31
好的,下面是一个简单的停车场管理系统的C++代码实现。
```cpp
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Car {
public:
Car(string license, string brand, string color, string type) :
m_license(license), m_brand(brand), m_color(color), m_type(type) {}
string getLicense() const { return m_license; }
string getBrand() const { return m_brand; }
string getColor() const { return m_color; }
string getType() const { return m_type; }
void setBrand(string brand) { m_brand = brand; }
void setColor(string color) { m_color = color; }
void setType(string type) { m_type = type; }
private:
string m_license; // 车牌号
string m_brand; // 车品牌
string m_color; // 车颜色
string m_type; // 车类型
};
class User {
public:
User(string username, string password, bool isAdmin) :
m_username(username), m_password(password), m_isAdmin(isAdmin) {}
bool isAdmin() const { return m_isAdmin; }
bool checkPassword(string password) const { return m_password == password; }
private:
string m_username; // 用户名
string m_password; // 密码
bool m_isAdmin; // 是否是管理员
};
class ParkingLot {
public:
void addCar(Car car) { m_cars.push_back(car); }
void removeCar(string license) {
for (auto it = m_cars.begin(); it != m_cars.end(); ++it) {
if (it->getLicense() == license) {
m_cars.erase(it);
break;
}
}
}
Car* findCar(string license) {
for (auto& car : m_cars) {
if (car.getLicense() == license) {
return &car;
}
}
return nullptr;
}
vector<Car>& getCars() { return m_cars; }
private:
vector<Car> m_cars; // 存储车辆信息
};
int main() {
ParkingLot parkingLot;
User user1("user1", "123", false); // 普通用户
User user2("admin", "admin", true); // 管理员
// 添加一些车辆信息
parkingLot.addCar(Car("粤A12345", "奔驰", "黑色", "轿车"));
parkingLot.addCar(Car("粤B67890", "宝马", "白色", "SUV"));
parkingLot.addCar(Car("粤C24680", "奥迪", "红色", "轿车"));
parkingLot.addCar(Car("粤D13579", "大众", "蓝色", "轿车"));
while (true) {
cout << "请输入用户名和密码:" << endl;
string username, password;
cin >> username >> password;
User* user = nullptr;
if (username == user1.getUsername() && user1.checkPassword(password)) {
user = &user1;
} else if (username == user2.getUsername() && user2.checkPassword(password)) {
user = &user2;
} else {
cout << "用户名或密码错误,请重新输入!" << endl;
continue;
}
cout << "欢迎使用停车场管理系统,您的权限为" << (user->isAdmin() ? "管理员" : "普通用户") << endl;
while (true) {
cout << "请选择操作:" << endl;
if (user->isAdmin()) {
cout << "1.添加车辆信息" << endl;
cout << "2.修改车辆信息" << endl;
cout << "3.删除车辆信息" << endl;
}
cout << "4.查看车辆信息" << endl;
cout << "5.退出" << endl;
int choice;
cin >> choice;
if (choice == 1 && user->isAdmin()) { // 添加车辆信息
string license, brand, color, type;
cout << "请输入车牌号、车品牌、车颜色、车类型:" << endl;
cin >> license >> brand >> color >> type;
parkingLot.addCar(Car(license, brand, color, type));
cout << "添加成功!" << endl;
} else if (choice == 2 && user->isAdmin()) { // 修改车辆信息
string license, brand, color, type;
cout << "请输入要修改的车牌号:" << endl;
cin >> license;
Car* car = parkingLot.findCar(license);
if (car == nullptr) {
cout << "未找到该车辆!" << endl;
} else {
cout << "请输入新的车品牌、车颜色、车类型:" << endl;
cin >> brand >> color >> type;
car->setBrand(brand);
car->setColor(color);
car->setType(type);
cout << "修改成功!" << endl;
}
} else if (choice == 3 && user->isAdmin()) { // 删除车辆信息
string license;
cout << "请输入要删除的车牌号:" << endl;
cin >> license;
parkingLot.removeCar(license);
cout << "删除成功!" << endl;
} else if (choice == 4) { // 查看车辆信息
cout << "车牌号\t车品牌\t车颜色\t车类型" << endl;
for (auto& car : parkingLot.getCars()) {
cout << car.getLicense() << "\t" << car.getBrand() << "\t" << car.getColor() << "\t" << car.getType() << endl;
}
} else if (choice == 5) { // 退出
return 0;
} else {
cout << "输入有误,请重新输入!" << endl;
}
}
}
return 0;
}
```
上述代码实现了停车场管理系统的基本功能,包括添加车辆信息、修改车辆信息、删除车辆信息和查看车辆信息等。普通用户只能查看车辆信息,而管理员可以添加、修改和删除车辆信息。
相关推荐
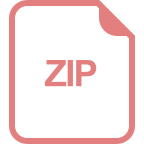
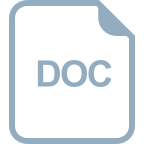
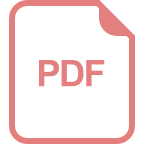














