多目标规划 Pareto前沿 Python实现
时间: 2024-05-15 13:16:34 浏览: 10
多目标规划是一种优化问题,其中需要同时优化多个目标函数。Pareto前沿是指在多目标优化问题中,所有不可改进的解的集合。在Pareto前沿上的所有解都是非支配的,即没有一个目标可以被单独优化,而不影响其他目标。在Python中,可以使用以下方法实现Pareto前沿:
1.定义目标函数:定义多个目标函数,例如f1(x)和f2(x)。
2.定义约束条件:定义多个约束条件,例如g1(x)和g2(x)。
3.定义优化问题:使用Scipy库中的minimize函数,定义多目标优化问题。
4.定义非支配排序函数:根据Pareto前沿的定义,需要对所有解进行非支配排序,以确定哪些解在Pareto前沿上。可以使用以下代码实现:
```
def nondominated_sorting(costs):
S=[[] for i in range(0,len(costs))]
front = [[]]
n=[0 for i in range(0,len(costs))]
for p in range(0,len(costs)):
S[p]=[]
n[p]=0
for q in range(0,len(costs)):
if costs[p][0]<costs[q][0] and costs[p][1]<costs[q][1]:
if q not in S[p]:
S[p].append(q)
elif costs[q][0]<costs[p][0] and costs[q][1]<costs[p][1]:
n[p] = n[p] + 1
if n[p]==0:
if p not in front[0]:
front[0].append(p)
i = 0
while(front[i] != []):
Q=[]
for p in front[i]:
for q in S[p]:
n[q] =n[q] - 1
if( n[q]==0):
if q not in Q:
Q.append(q)
i = i + 1
front.append(Q)
del front[len(front)-1]
return front
```
5.定义Pareto前沿函数:根据非支配排序的结果,可以定义一个函数来计算Pareto前沿。以下是一个例子:
```
def calculate_pareto_front(costs):
fronts = nondominated_sorting(costs)
pareto_front = []
for front in fronts:
for i in front:
pareto_front.append(costs[i])
return pareto_front
```
6.运行优化问题:使用定义的优化问题和Pareto前沿函数,可以计算Pareto前沿。以下是一个例子:
```
from scipy.optimize import minimize
import numpy as np
def f1(x):
return x[0]**2
def f2(x):
return (x[0]-2)**2
def g1(x):
return x[0] - 1
def g2(x):
return 2 - x[0]
def objective(x):
return [f1(x), f2(x)]
def constraint1(x):
return g1(x)
def constraint2(x):
return g2(x)
x0 = [0.5]
bounds = [(0, 2)]
constraints = [{'type': 'ineq', 'fun': constraint1}, {'type': 'ineq', 'fun': constraint2}]
result = minimize(objective, x0, method='SLSQP', bounds=bounds, constraints=constraints)
pareto_front = calculate_pareto_front([[f1(x), f2(x)] for x in result.x])
print(pareto_front)
```
在这个例子中,定义了两个目标函数f1和f2,以及两个约束条件g1和g2。使用Scipy库中的minimize函数,定义了多目标优化问题。然后,使用定义的非支配排序和Pareto前沿函数计算Pareto前沿。最后,打印出Pareto前沿的结果。
相关推荐
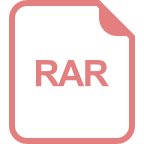














