std::string::npos
时间: 2023-09-17 15:13:31 浏览: 41
`std::string::npos` 是一个字符串的静态成员,表示一个无效的或者不存在的位置。在 C++ 中,它通常被用来表示字符串查找函数(如 `find()`)未找到匹配项的情况。`std::string::npos` 的值通常是 -1,因为它是一个无符号整数类型,因此 -1 被解释为最大可能的值。
相关问题
std::string::npos什么意思
`std::string::npos` 是 C++ 标准库中 `std::string` 类的静态成员变量,它表示字符串的无效或不存在的位置。
具体来说,`std::string::npos` 是一个特殊的静态成员变量,它的值是一个大于或等于 `std::string` 类型中的任何可能索引值的常量。通常情况下,它被定义为 `-1` 或一个足够大的整数值。
在字符串操作中,当使用 `std::string` 的成员函数(例如 `find()`、`rfind()`、`find_first_of()`、`find_last_of()` 等)进行查找操作时,如果没有找到指定的子字符串或字符,这些函数会返回 `std::string::npos`。这样,我们就可以通过检查返回值是否等于 `std::string::npos` 来判断是否找到了目标。
简而言之,`std::string::npos` 表示字符串中某个位置的索引值不存在或无效。在字符串查找操作中,它用于指示未找到目标子字符串或字符。
std::string::npos是什么
`std::string::npos`是`std::string`类的静态成员,它表示一个特殊的无效位置。
在C++中,当使用`std::string`的成员函数`find()`时,如果没有找到指定的子字符串或字符,它将返回一个特殊的值`std::string::npos`。这个值是一个常量,其类型是`std::string::size_type`,通常是一个无符号整数。
`std::string::npos`的值在不同的实现中可能不同,但通常它被定义为一个非法的字符串位置,用于表示搜索失败。
因此,当我们使用`find()`函数搜索一个子字符串或字符并检查返回值是否等于`std::string::npos`时,我们可以判断是否成功找到了指定的子字符串或字符。
示例用法:
```cpp
std::string str = "Hello, World!";
size_t pos = str.find("World"); // 查找子字符串"World"
if (pos != std::string::npos) {
std::cout << "Found at position: " << pos << std::endl;
} else {
std::cout << "Not found" << std::endl;
}
```
在上述示例中,如果找到了子字符串"World",则输出它在原始字符串中的位置;否则,输出"Not found"。
希望能解答您的疑问,如果还有其他问题,请随时提问。
相关推荐
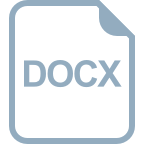
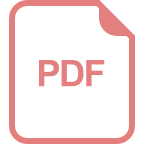
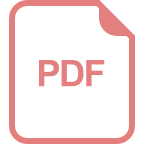








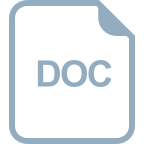
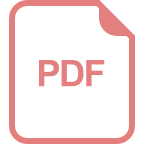
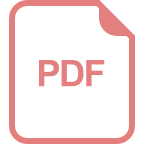
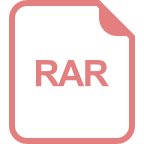
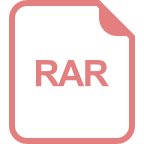