C语言已知数列{1,3,5,9,23},完成以下操作 1)用链表保存该数列 2)在链表中删除一个元素(如删除3) 建议: 1. 设计创建空链表函数 2. 设计删除一个元素的函代码
时间: 2024-03-12 09:46:10 浏览: 17
以下是一个使用链表保存数列、删除指定元素的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct node {
int data;
struct node* next;
};
// 创建空链表函数
struct node* create_list(int data) {
struct node* head = (struct node*) malloc(sizeof(struct node));
head->data = data;
head->next = NULL;
return head;
}
// 在链表末尾添加元素函数
void add_to_list(struct node* head, int data) {
struct node* p = head;
while (p->next != NULL) {
p = p->next;
}
struct node* node = (struct node*) malloc(sizeof(struct node));
node->data = data;
node->next = NULL;
p->next = node;
}
// 删除元素函数
void delete_from_list(struct node* head, int data) {
struct node* p = head;
while (p->next != NULL) {
if (p->next->data == data) {
struct node* tmp = p->next;
p->next = p->next->next;
free(tmp);
return;
}
p = p->next;
}
}
// 打印链表函数
void print_list(struct node* head) {
struct node* p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
// 创建空链表
struct node* head = create_list(1);
// 在链表末尾添加元素
add_to_list(head, 3);
add_to_list(head, 5);
add_to_list(head, 9);
add_to_list(head, 23);
// 打印链表
printf("原始链表:");
print_list(head);
// 删除元素
delete_from_list(head, 3);
// 打印链表
printf("删除元素后的链表:");
print_list(head);
return 0;
}
```
这个示例代码中,首先定义了一个链表节点结构体 `struct node`,包含了数据和指向下一个节点的指针两个属性。然后定义了创建空链表函数 `create_list`,参数为链表的第一个节点的数据,函数返回指向链表头节点的指针。定义了在链表末尾添加元素的函数 `add_to_list`,参数为链表头节点的指针和要添加的元素值。定义了删除元素的函数 `delete_from_list`,参数为链表头节点的指针和要删除的元素值。最后定义了打印链表的函数 `print_list`,参数为链表头节点的指针。在 `main` 函数中,先创建空链表,然后在链表末尾添加元素,打印链表,删除元素,再打印链表。
相关推荐
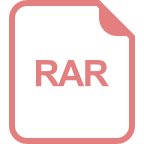
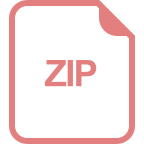














