#include<iostream> #include<cmath> using namespace std; int abs(int x) {int b; if(x>=0){b=x; }else{b=-x; } cout<<b; return 0; } float abs(float x) {float b; if(x>=0){b=x; }else{b=-x; } cout<<b; return 0; } double abs(double x) {double b; if(x>=0){b=x; }else{b=-x; } cout<<b; return 0; } void main() {int x=-10; float y=-2.5; double z=-3.8; cout<<abs(x)<<endl<<abs(y)<<endl<<abs(z)<<endl; return 0
时间: 2024-02-19 18:25:33 浏览: 17
这是一个使用函数重载实现绝对值计算的 C++ 程序。其中,abs 函数被重载了三次,分别用于计算 int、float 和 double 类型的绝对值。在函数内部,通过条件语句判断参数 x 的正负性,然后分别赋值给变量 b,并输出 b 的值。最后,程序在主函数中调用三次不同类型的 abs 函数,并输出结果。
相关问题
为以下代码定义窗口函数#include <iostream> #include <iomanip> #include <string> using namespace std; int main() {
要为这个代码定义窗口函数,需要知道窗口函数的具体要求是什么。通常情况下,窗口函数是一种在信号处理中使用的数学函数,它可以用来改变信号的频率分布或者减小信号的波动性。在编程中,窗口函数通常被用来处理频域数据。如果你需要在这个代码中定义一个窗口函数,可以考虑使用C++中的数学库,比如cmath库中的函数,或者自己编写一个窗口函数。具体实现方法会根据窗口函数的要求而有所不同。
#include<iostream> #include<algorithm> #include<iomanip> #include<cmath> #include<cstdio> #include<cstring> #include<string> using namespace std; int main() { int count = 0; string a; getline(cin, a); for (int i = 0; i < a.size(); ++i) { /*if (a[i] >= 'A' && a[i] <= 'Z') count++; if (a[i] >= 'a' && a[i] <= 'z') count++; if (a[i] >= '0' && a[i] <= '9') count++;*/ if (a[i] != ' ' && a[i] != '\n') ++count; } cout << count; return 0; }给上面的代码加上注释
```
#include<iostream> // 输入输出流
#include<algorithm> // 算法库
#include<iomanip> // 输入输出格式控制库
#include<cmath> // 数学库
#include<cstdio> // 标准输入输出库
#include<cstring> // 字符串库
#include<string> // 字符串库
using namespace std; // 标准命名空间
int main() { // 主函数开始
int count = 0; // 定义变量count并初始化为0
string a; // 定义字符串变量a
getline(cin, a); // 从标准输入流中读取整行字符串存入变量a中
for (int i = 0; i < a.size(); ++i) // 循环遍历字符串a中的每一个字符
{
/*if (a[i] >= 'A' && a[i] <= 'Z') // 如果字符是大写字母
count++; // 计数器加1
if (a[i] >= 'a' && a[i] <= 'z') // 如果字符是小写字母
count++; // 计数器加1
if (a[i] >= '0' && a[i] <= '9') // 如果字符是数字
count++; // 计数器加1*/
if (a[i] != ' ' && a[i] != '\n') // 如果字符不是空格或换行符
++count; // 计数器加1
}
cout << count; // 输出计数器的值
return 0; // 返回0表示程序正常结束
} // 主函数结束
```
相关推荐
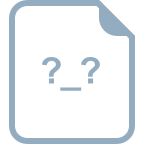
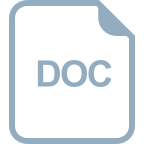
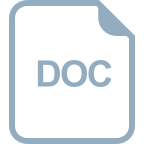














