python如何爬取视频资源
时间: 2023-03-27 08:05:07 浏览: 83
可以使用 Python 中的 requests 库和 BeautifulSoup 库来爬取视频资源。首先,使用 requests 库发送 HTTP 请求获取网页源代码,然后使用 BeautifulSoup 库解析网页源代码,找到视频资源的链接,最后使用 requests 库下载视频资源。具体实现可以参考相关的 Python 爬虫教程。
相关问题
python爬取电影视频
爬取电影视频的过程分为两个主要步骤:获取电影资源的链接和下载电影视频。
获取电影资源链接可以通过以下方法之一:
1. 使用 Python 的网络爬虫库(如 requests、BeautifulSoup、Scrapy)访问电影网站,解析网页内容,提取电影资源的链接。
2. 如果目标网站提供了 API 接口,可以通过调用接口获取电影资源链接。
3. 模拟用户操作,使用自动化测试工具(如 Selenium)打开网页,搜索电影,获取链接。
下载电影视频可以使用 Python 的下载库(如 requests、urllib)来实现。获取到电影资源链接后,你可以使用这些库发送请求并下载视频文件到本地。
需要注意的是,尊重版权是非常重要的。确保你在爬取电影视频时遵循相关法律法规,并且只从合法授权的渠道获取电影资源。
python 爬取网站视频代码
### 回答1:
下面是一个简单的 Python 代码爬取网站视频的例子:
```
import requests
url = "http://example.com/video.mp4"
response = requests.get(url)
with open("video.mp4", "wb") as f:
f.write(response.content)
```
这段代码使用了 `requests` 库来发送 HTTP 请求,并将响应内容写入到本地文件 `video.mp4` 中。
请注意,爬取网站视频可能违反网站的版权政策,并且不合法。请务必尊重网站的政策并遵守相关的法律法规。
### 回答2:
要用Python爬取网站视频,你可以使用第三方库(例如requests和beautifulsoup)来处理HTTP请求和解析HTML。
首先,你需要使用requests库发送HTTP请求获取网页的源代码。你可以使用requests.get()方法,并将目标网页URL作为参数传递给该方法。然后,你可以使用response.text属性来获取网页的原始HTML代码。
接下来,你需要使用beautifulsoup库来解析HTML代码,以便找到视频的URL。你可以使用BeautifulSoup()函数,并将之前获得的网页代码作为参数传递给它。然后,你可以使用CSS选择器或XPath表达式在网页中找到视频元素。例如,如果视频元素具有特定的标签、类或ID,你可以使用.select()方法或.find()方法来找到它。之后,你可以使用.get()方法来获取视频元素的URL属性。
一旦你获得了视频的URL,你可以使用requests库下载视频。你可以使用requests.get()方法,并将视频的URL作为参数传递给该方法。然后,你可以使用response.content属性获取视频的二进制数据。
最后,你可以使用Python来保存视频文件。你可以使用open()函数创建一个新文件,然后使用.write()方法将二进制数据写入该文件。记得关闭文件以释放资源。
以上就是使用Python爬取网站视频的基本步骤。当然,在实际应用中,你可能需要处理一些异常情况,例如处理缓存、验证、登录等。此外,在下载视频时,请确保你遵循了相关网站的使用条款和法律法规。
相关推荐
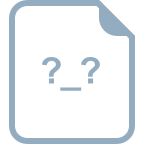
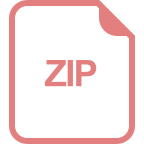
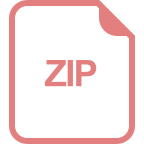












