图书馆新进一批图书,每本图书的名字均不超过50个字符。编写一程序,将这些图书的名字按照从小到大的顺序排列。要求 排序通过函数进行,数据的输入输出及函数调用在主函数中进行 c语言 void sort
时间: 2024-03-08 19:48:39 浏览: 51
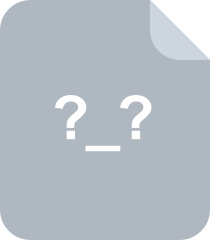
C语言程序设计-编写函数用选择排序法对数组中的数据进行从小到大的排序;

可以使用冒泡排序或快速排序来实现这个功能。
冒泡排序的代码如下:
```c
void bubble_sort(char books[][51], int n) {
int i, j;
char tmp[51];
for (i = 0; i < n-1; ++i) {
for (j = 0; j < n-i-1; ++j) {
if (strcmp(books[j], books[j+1]) > 0) {
strcpy(tmp, books[j]);
strcpy(books[j], books[j+1]);
strcpy(books[j+1], tmp);
}
}
}
}
```
快速排序的代码如下:
```c
void quick_sort(char books[][51], int left, int right) {
if (left >= right) return;
int i = left, j = right;
char tmp[51];
char pivot[51];
strcpy(pivot, books[(left+right)>>1]);
while (i <= j) {
while (strcmp(books[i], pivot) < 0) ++i;
while (strcmp(books[j], pivot) > 0) --j;
if (i <= j) {
strcpy(tmp, books[i]);
strcpy(books[i], books[j]);
strcpy(books[j], tmp);
++i, --j;
}
}
quick_sort(books, left, j);
quick_sort(books, i, right);
}
```
这两个函数都可以在主函数中调用,例如:
```c
int main() {
char books[100][51];
int n, i;
scanf("%d", &n);
for (i = 0; i < n; ++i) {
scanf("%s", books[i]);
}
//bubble_sort(books, n);
quick_sort(books, 0, n-1);
for (i = 0; i < n; ++i) {
printf("%s\n", books[i]);
}
return 0;
}
```
注意,这里使用了一个二维字符数组来存储图书的名字,因为每本图书的名字均不超过50个字符。在排序函数中,使用了strcmp函数来比较两个字符串的大小关系。在快速排序函数中,使用了递归的方式来实现快速排序。
阅读全文
相关推荐
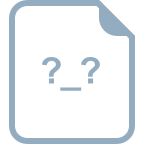
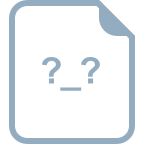















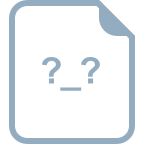