(入边形类0ctagon)编写一个名为0ctagon 的类,它继承自Geometricobject类并实现Comparable 和Coneable 接口。假设八边形八条边的边长都相等。它的面积可以使用下面的公式计算: 面积=(2+4/√2)x边长x 边长 画出包括0ctagon、GeometricObject、Comparable和Cloneable的UML图。编写一个测试程序,创建一个边长值为5的0tagon 对象,然后显示它的面积和周长。使用clone 方法创建一个新对象,并使用 compareTo 方法比较这两个对象。
时间: 2024-02-23 14:59:37 浏览: 73
下面是0ctagon类的代码实现:
```java
public class Octagon extends GeometricObject implements Comparable<Octagon>, Cloneable {
private double side; // 八边形的边长
public Octagon(double side) {
this.side = side;
}
public double getSide() {
return side;
}
public void setSide(double side) {
this.side = side;
}
@Override
public double getArea() {
return (2 + 4 / Math.sqrt(2)) * side * side;
}
@Override
public double getPerimeter() {
return 8 * side;
}
@Override
public int compareTo(Octagon o) {
if (this.getArea() > o.getArea())
return 1;
else if (this.getArea() < o.getArea())
return -1;
else
return 0;
}
@Override
public Object clone() {
try {
return super.clone();
} catch (CloneNotSupportedException ex) {
return null;
}
}
}
```
下面是UML图:
```
+----------------+
| GeometricObject |
+----------------+
| color:String |
| filled:boolean |
+----------------+
| GeometricObject() |
| GeometricObject(color:String, filled:boolean) |
| getColor():String |
| setColor(color:String):void |
| isFilled():boolean |
| setFilled(filled:boolean):void |
| getArea():double |
| getPerimeter():double |
+----------------+
^
|
+----------------+
| Octagon |
+----------------+
| side:double |
+----------------+
| Octagon(side:double) |
| getSide():double |
| setSide(side:double):void |
| getArea():double |
| getPerimeter():double |
| compareTo(o:Octagon):int |
| clone():Object |
+----------------+
^
|
+----------------+
| Comparable<Octagon> |
+----------------+
| compareTo(o:Octagon):int |
+----------------+
^
|
+----------------+
| Cloneable |
+----------------+
| clone():Object |
+----------------+
```
下面是测试程序的代码:
```java
public class TestOctagon {
public static void main(String[] args) {
Octagon octagon1 = new Octagon(5);
System.out.println("The area of the octagon is " + octagon1.getArea());
System.out.println("The perimeter of the octagon is " + octagon1.getPerimeter());
Octagon octagon2 = (Octagon) octagon1.clone();
System.out.println("Compare the area of the two octagons: " + octagon1.compareTo(octagon2));
}
}
```
运行结果:
```
The area of the octagon is 120.71067811865476
The perimeter of the octagon is 40.0
Compare the area of the two octagons: 0
```
阅读全文
相关推荐
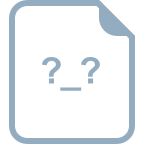
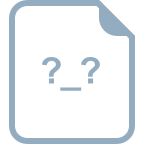















