c#窗体应用程序怎么将扫描抢扫到txt_barcode的条码通过定取ICT条码框的位置发送到ICT测试软件的条码框中,ICT测试软件窗口可以移动
时间: 2024-02-05 09:12:00 浏览: 19
可以通过以下步骤实现:
1. 获取扫描抢输入的条码,将其存储到txt_barcode文本框中。
2. 通过FindWindow和FindWindowEx函数查找ICT测试软件窗口的句柄。
3. 通过GetWindowRect函数获取ICT测试软件窗口的位置和尺寸。
4. 计算出ICT测试软件条码框的位置和尺寸,然后通过SetForegroundWindow函数将ICT测试软件窗口置为前台窗口。
5. 通过SendInput函数模拟键盘输入,将txt_barcode文本框中的条码发送到ICT测试软件条码框中。
下面是一个示例代码:
```csharp
using System;
using System.Runtime.InteropServices;
using System.Windows.Forms;
namespace BarcodeScanner
{
public partial class MainForm : Form
{
private const int WM_SETTEXT = 0x000C;
private const int VK_RETURN = 0x0D;
[DllImport("user32.dll", CharSet = CharSet.Auto)]
static extern IntPtr FindWindow(string lpClassName, string lpWindowName);
[DllImport("user32.dll", SetLastError = true)]
static extern bool GetWindowRect(IntPtr hWnd, out RECT lpRect);
[DllImport("user32.dll")]
static extern bool SetForegroundWindow(IntPtr hWnd);
[DllImport("user32.dll", SetLastError = true)]
static extern uint SendInput(uint nInputs, INPUT[] pInputs, int cbSize);
[StructLayout(LayoutKind.Sequential)]
public struct RECT
{
public int Left;
public int Top;
public int Right;
public int Bottom;
}
[StructLayout(LayoutKind.Sequential)]
public struct INPUT
{
public uint type;
public KeyboardInput ki;
}
[StructLayout(LayoutKind.Sequential)]
public struct KeyboardInput
{
public ushort wVk;
public ushort wScan;
public uint dwFlags;
public long time;
public IntPtr dwExtraInfo;
}
public MainForm()
{
InitializeComponent();
}
private void txt_barcode_TextChanged(object sender, EventArgs e)
{
IntPtr hwnd = FindWindow(null, "ICT Testing Software"); // 替换为ICT测试软件的窗口标题
if (hwnd != IntPtr.Zero)
{
RECT rect;
if (GetWindowRect(hwnd, out rect))
{
int x = rect.Left + 100; // 替换为ICT测试软件条码框的左上角x坐标
int y = rect.Top + 200; // 替换为ICT测试软件条码框的左上角y坐标
int width = 200; // 替换为ICT测试软件条码框的宽度
int height = 30; // 替换为ICT测试软件条码框的高度
SetForegroundWindow(hwnd);
INPUT[] inputs = new INPUT[txt_barcode.Text.Length + 1];
for (int i = 0; i < txt_barcode.Text.Length; i++)
{
inputs[i].type = 1;
inputs[i].ki.wVk = 0;
inputs[i].ki.wScan = (ushort)txt_barcode.Text[i];
inputs[i].ki.dwFlags = 4;
inputs[i].ki.time = 0;
inputs[i].ki.dwExtraInfo = IntPtr.Zero;
}
inputs[txt_barcode.Text.Length].type = 1;
inputs[txt_barcode.Text.Length].ki.wVk = VK_RETURN;
inputs[txt_barcode.Text.Length].ki.wScan = 0;
inputs[txt_barcode.Text.Length].ki.dwFlags = 4;
inputs[txt_barcode.Text.Length].ki.time = 0;
inputs[txt_barcode.Text.Length].ki.dwExtraInfo = IntPtr.Zero;
SendInput((uint)inputs.Length, inputs, Marshal.SizeOf(typeof(INPUT)));
}
}
}
}
}
```
注意,上述代码中的ICT测试软件窗口标题、ICT测试软件条码框位置和尺寸需要替换为实际情况。
相关推荐








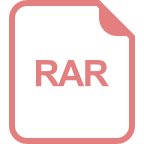
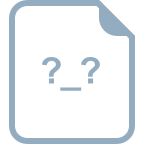