datagridview导入导出excel
时间: 2023-06-05 19:47:40 浏览: 251
如何在DataGridView中导入导出Excel?
1. 导入Excel:
可以通过以下步骤将Excel数据导入到DataGridView中:
- 首先,需要使用.NET的Excel对象模型(如Microsoft.Office.Interop.Excel)来打开Excel文件。
- 接着,需要选择要读取的工作表,并使用循环遍历所有单元格。
- 最后,将单元格中的数据存储到一个数组或List中,并将其绑定到DataGridView中。
2. 导出Excel:
可以通过以下步骤将DataGridView中的数据导出到Excel中:
- 首先,需要创建一个空的Excel文件。
- 接着,需要选择要写入数据的工作表,并使用循环遍历DataGridView中的所有行和列。
- 最后,将每个单元格的数据写入到对应的Excel单元格中,并保存Excel文件。
相关问题
c#datagridview 导入和导出excel
在 C# 中,您可以使用以下代码实现 DataGridView 数据的导入和导出 Excel 文件的功能。
导入 Excel 文件到 DataGridView:
```csharp
using System;
using System.Data;
using System.Windows.Forms;
using Excel = Microsoft.Office.Interop.Excel;
public void ImportExcelToDataGridView(DataGridView dataGridView, string filePath)
{
Excel.Application excelApp = new Excel.Application();
Excel.Workbook workbook = excelApp.Workbooks.Open(filePath);
Excel.Worksheet worksheet = workbook.ActiveSheet;
int rowCount = worksheet.UsedRange.Rows.Count;
int columnCount = worksheet.UsedRange.Columns.Count;
DataTable dataTable = new DataTable();
for (int col = 1; col <= columnCount; col++)
{
dataTable.Columns.Add(worksheet.Cells[1, col].Value.ToString());
}
for (int row = 2; row <= rowCount; row++)
{
DataRow dataRow = dataTable.NewRow();
for (int col = 1; col <= columnCount; col++)
{
dataRow[col - 1] = worksheet.Cells[row, col].Value;
}
dataTable.Rows.Add(dataRow);
}
dataGridView.DataSource = dataTable;
workbook.Close();
excelApp.Quit();
}
```
导出 DataGridView 数据到 Excel 文件:
```csharp
using System;
using System.Data;
using System.Windows.Forms;
using Excel = Microsoft.Office.Interop.Excel;
public void ExportDataGridViewToExcel(DataGridView dataGridView, string filePath)
{
Excel.Application excelApp = new Excel.Application();
Excel.Workbook workbook = excelApp.Workbooks.Add();
Excel.Worksheet worksheet = workbook.ActiveSheet;
for (int col = 1; col <= dataGridView.Columns.Count; col++)
{
worksheet.Cells[1, col] = dataGridView.Columns[col - 1].HeaderText;
worksheet.Cells[1, col].Font.Bold = true;
}
for (int row = 0; row < dataGridView.Rows.Count; row++)
{
for (int col = 0; col < dataGridView.Columns.Count; col++)
{
worksheet.Cells[row + 2, col + 1] = dataGridView.Rows[row].Cells[col].Value.ToString();
}
}
worksheet.Columns.AutoFit();
workbook.SaveAs(filePath);
workbook.Close();
excelApp.Quit();
}
```
您可以调用 `ImportExcelToDataGridView` 方法将 Excel 文件导入到 DataGridView,传入要导入的 DataGridView 对象和 Excel 文件路径。调用 `ExportDataGridViewToExcel` 方法将 DataGridView 数据导出到 Excel 文件,传入要导出的 DataGridView 对象和 Excel 文件路径。
请注意,导入和导出 Excel 文件需要在项目中引用 Microsoft.Office.Interop.Excel 库。
阅读全文
相关推荐
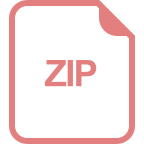
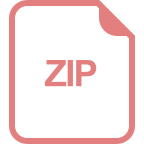
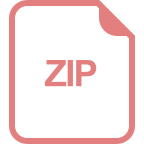
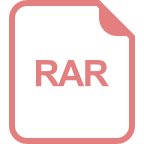
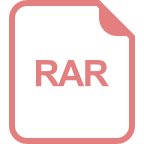
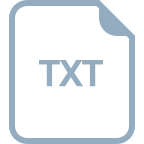
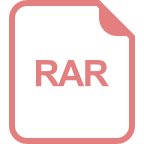
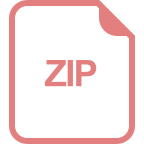
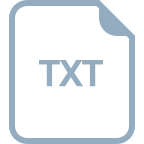
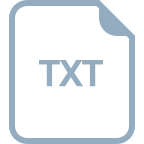
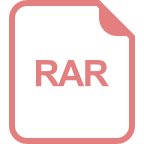
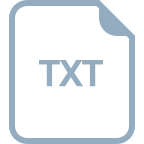