写出 superpoint加入旋转不变性的代码
时间: 2023-10-26 21:04:51 浏览: 185
以下是一个简单示例,展示了如何在SuperPoint中加入旋转不变性的代码:
```python
import cv2
import numpy as np
import torch
import torchvision.transforms as transforms
from superpoint.models import SuperPointNet
# 创建 SuperPoint 模型实例
superpoint = SuperPointNet()
# 加载预训练权重
superpoint.load_state_dict(torch.load('superpoint_weights.pth'))
superpoint.eval()
# 定义图像预处理函数
def preprocess_image(image):
# 将图像转为灰度图
image_gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 将图像转为浮点数类型
image_gray = image_gray.astype(np.float32) / 255.0
# 转为PyTorch张量
image_tensor = transforms.ToTensor()(image_gray)
# 调整维度为 [batch_size, channels, height, width]
image_tensor = image_tensor.unsqueeze(0)
return image_tensor
# 定义旋转函数
def rotate_image(image, angle):
height, width = image.shape[:2]
# 计算旋转中心
center = (width / 2, height / 2)
# 获取旋转矩阵
rotation_matrix = cv2.getRotationMatrix2D(center, angle, 1.0)
# 执行旋转操作
rotated_image = cv2.warpAffine(image, rotation_matrix, (width, height))
return rotated_image
# 加载图像
image = cv2.imread('image.jpg')
# 图像预处理
image_tensor = preprocess_image(image)
# 提取特征点和描述子
keypoints, descriptors = superpoint(image_tensor)
# 将图像旋转 45 度
rotated_image = rotate_image(image, 45)
# 图像预处理
rotated_image_tensor = preprocess_image(rotated_image)
# 提取旋转后的图像的特征点和描述子
rotated_keypoints, rotated_descriptors = superpoint(rotated_image_tensor)
# 进行匹配或其他后续处理
# ...
```
请注意,上述代码仅用于演示目的,实际上,要加入旋转不变性,还需要根据具体的需求进行适当的调整和优化。
阅读全文
相关推荐
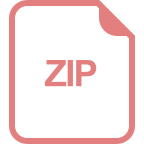
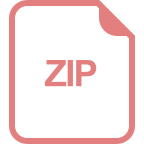

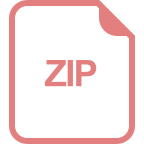
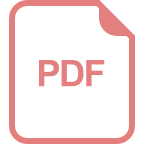
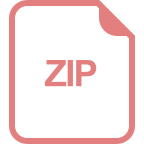
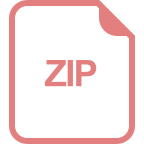
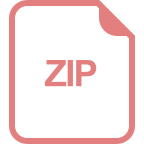
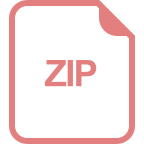
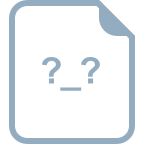
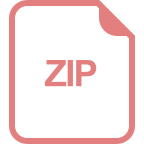
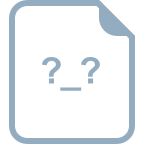
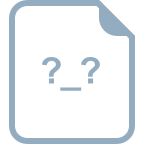
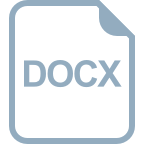
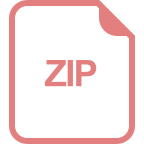