分析以下代码思路package s5; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; public class Sy5_2 { public static void main(String[] args) { try { // 创建FileInputStream对象读取输入文件 FileInputStream fis = new FileInputStream("myInputfile.txt"); // 创建FileOutputStream对象写入输出文件 FileOutputStream fos = new FileOutputStream("myOutputfile.txt"); // 读取输入文件并写入输出文件 int content; while ((content = fis.read()) != -1) { fos.write(content); } // 关闭流 fis.close(); fos.close(); } catch (IOException e) { e.printStackTrace(); } } }
时间: 2024-02-14 18:22:01 浏览: 28
这段代码的主要思路是将一个文件的内容读取出来并写入到另一个文件中。
具体分析如下:
1. 第一行代码指定了代码所在的包名为s5。
2. 第二行到第四行依次引入了Java的三个IO类:FileInputStream、FileOutputStream和IOException。
3. 第五行定义了一个名为Sy5_2的公共类(public class),这个类包含了一个名为main的静态方法(public static void main(String[] args))。
4. 在main方法中,首先创建了一个FileInputStream对象,该对象的参数为myInputfile.txt,即要读取的文件名。
5. 然后创建了一个FileOutputStream对象,该对象的参数为myOutputfile.txt,即要写入的文件名。
6. 接下来使用while循环,读取输入文件的内容并写入到输出文件中。循环的条件是当读取的内容不为-1时继续循环,-1表示已经读到文件末尾。
7. 最后关闭输入流和输出流,释放资源。如果发生异常,则打印异常信息。
需要注意的是,这段代码中的异常处理比较简单,只是简单地打印了异常信息,并没有进行具体的处理。在实际开发中,应该根据具体情况进行更加详细的异常处理。同时,如果要读取和写入的文件内容较大,建议使用缓冲流进行处理,可以提高读写性能。
相关问题
package src; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.BufferedInputStream; import java.io.BufferedOutputStream; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import javax.swing.JButton; import javax.swing.JFileChooser; import javax.swing.JFrame; import javax.swing.JOptionPane; import javax.swing.JPanel; import javax.swing.JScrollPane; import javax.swing.JTextArea; public class Test3 extends JFrame { JTextArea txta=new JTextArea(10,21); JScrollPane scrp=new JScrollPane(txta); JButton btn1=new JButton("读t3_1.txt到文本框"); JButton btn2=new JButton("保存到t3_2.txt"); JPanel p=new JPanel(); public Test3() { p.add(scrp); p.add(btn1); p.add(btn2); this.setContentPane(p); this.setSize(300,270); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); this.setLocationRelativeTo(null); this.setVisible(true); } public static void main(String[] args) { Test3 fr=new Test3(); fr.setTitle("第3题"); } }补全上述代码
可以按照以下步骤进行代码的补全:
1. 在Test3类中添加一个构造方法,然后在方法中为btn1和btn2添加监听器,用于实现读取和保存文件的功能。
2. 在读取文件的监听器中,可以使用JFileChooser组件选择文件,然后使用FileInputStream和BufferedInputStream读取文件内容,并将内容显示在文本框中。
3. 在保存文件的监听器中,可以使用JFileChooser组件选择保存文件的路径和文件名,然后使用FileOutputStream和BufferedOutputStream将文本框中的内容写入到文件中。
完整代码如下:
```
package src;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
public class Test3 extends JFrame {
JTextArea txta = new JTextArea(10, 21);
JScrollPane scrp = new JScrollPane(txta);
JButton btn1 = new JButton("读t3_1.txt到文本框");
JButton btn2 = new JButton("保存到t3_2.txt");
JPanel p = new JPanel();
public Test3() {
p.add(scrp);
p.add(btn1);
p.add(btn2);
this.setContentPane(p);
this.setSize(300, 270);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setLocationRelativeTo(null);
this.setVisible(true);
// 为btn1添加监听器,读取文件内容
btn1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// 创建文件选择器
JFileChooser chooser = new JFileChooser();
chooser.setDialogTitle("选择文件");
int result = chooser.showOpenDialog(Test3.this);
if (result == JFileChooser.APPROVE_OPTION) {
try {
// 读取文件内容
File file = chooser.getSelectedFile();
FileInputStream fis = new FileInputStream(file);
BufferedInputStream bis = new BufferedInputStream(fis);
byte[] buffer = new byte[(int) file.length()];
bis.read(buffer);
String text = new String(buffer);
txta.setText(text);
bis.close();
fis.close();
} catch (IOException ex) {
JOptionPane.showMessageDialog(Test3.this, "读取文件失败");
}
}
}
});
// 为btn2添加监听器,保存文件内容
btn2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// 创建文件选择器
JFileChooser chooser = new JFileChooser();
chooser.setDialogTitle("保存文件");
int result = chooser.showSaveDialog(Test3.this);
if (result == JFileChooser.APPROVE_OPTION) {
try {
// 写入文件内容
File file = chooser.getSelectedFile();
FileOutputStream fos = new FileOutputStream(file);
BufferedOutputStream bos = new BufferedOutputStream(fos);
String text = txta.getText();
byte[] buffer = text.getBytes();
bos.write(buffer);
bos.close();
fos.close();
} catch (IOException ex) {
JOptionPane.showMessageDialog(Test3.this, "保存文件失败");
}
}
}
});
}
public static void main(String[] args) {
Test3 fr = new Test3();
fr.setTitle("第3题");
}
}
```
java: 不兼容的类型: java.io.FileInputStream无法转换为java.lang.String
这个错误是因为程序试图将一个文件输入流对象(java.io.FileInputStream)转换为字符串类型(java.lang.String),这两种类型是不兼容的,因此会出现错误。
要解决这个问题,您需要使用文件输入流对象的方法来读取文件的内容并将其转换为字符串,例如使用Scanner或BufferedReader等类来读取文件。以下是使用Scanner读取文件并将其转换为字符串的示例代码:
```
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class FileToString {
public static void main(String[] args) {
String fileName = "example.txt";
try {
File file = new File(fileName);
Scanner scanner = new Scanner(file);
String fileContent = scanner.useDelimiter("\\Z").next();
scanner.close();
System.out.println(fileContent);
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
```
这个示例代码中,我们首先创建一个File对象来指定要读取的文件,然后使用Scanner类来读取文件内容。使用useDelimiter("\\Z").next()方法可以将整个文件读取为一个字符串对象。最后,我们将读取到的字符串打印出来。
注意:在使用Scanner读取文件时,必须确保文件存在并且可以被读取。如果文件不存在或者没有读取权限,会抛出FileNotFoundException异常。
相关推荐
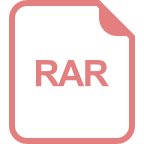
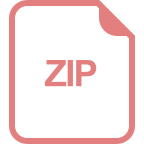
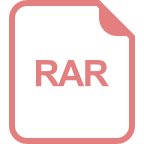












