onnx模型部署flask,在flask网络上提交测试图片,并将结果呈现在flask网页上,请注意代码的严谨性
时间: 2024-05-03 11:21:49 浏览: 7
以下是一个基本的ONNX模型部署Flask应用程序的示例代码:
```python
from flask import Flask, render_template, request
import numpy as np
import onnxruntime as ort
app = Flask(__name__)
# 加载ONNX模型
session = ort.InferenceSession("model.onnx")
input_name = session.get_inputs()[0].name
output_name = session.get_outputs()[0].name
@app.route('/')
def index():
return render_template('index.html')
@app.route('/predict', methods=['POST'])
def predict():
# 获取上传的图片文件
file = request.files['image']
# 将图片转换为numpy数组
image = np.frombuffer(file.read(), np.uint8)
image = cv2.imdecode(image, cv2.IMREAD_COLOR)
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
image = cv2.resize(image, (224, 224))
image = np.array(image).astype(np.float32)
image = np.transpose(image, (2, 0, 1))
image = np.expand_dims(image, axis=0)
# 运行模型进行预测
outputs = session.run([output_name], {input_name: image})
result = np.argmax(outputs)
# 返回预测结果
if result == 0:
return "Cat"
else:
return "Dog"
if __name__ == '__main__':
app.run(debug=True)
```
该示例代码中,首先加载了ONNX模型并创建了一个Flask应用程序。然后,定义了一个主页(index)和一个预测处理(predict)的路由。在预测处理路由中,首先获取上传的图片文件,并将其转换为numpy数组。然后,将图像传递给ONNX模型进行预测。最后,根据预测结果返回“Cat”或“Dog”字符串。
需要注意的是,在实际部署ONNX模型时,可能需要对输入和输出进行一些额外的处理,以确保模型能够正确地运行。此外,还需要考虑模型的性能和安全性,以保证应用程序的可靠性和稳定性。
相关推荐
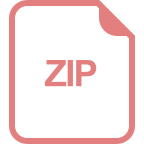














