Android 查询数据库后将数据以json形式传入POST请求接口进行发送
时间: 2024-05-12 12:19:18 浏览: 18
以下是一个简单的示例代码,假设你已经查询了名为“table_name”的数据库,并将结果存储在一个名为“cursor”的游标对象中:
```
// 创建一个空的 JSON 数组
JSONArray jsonArray = new JSONArray();
// 遍历游标中的数据
while (cursor.moveToNext()) {
// 为每一行数据创建一个 JSON 对象
JSONObject jsonObject = new JSONObject();
try {
// 将每一列数据添加到 JSON 对象中
jsonObject.put("column_name_1", cursor.getString(cursor.getColumnIndex("column_name_1")));
jsonObject.put("column_name_2", cursor.getString(cursor.getColumnIndex("column_name_2")));
// ...
// 将 JSON 对象添加到 JSON 数组中
jsonArray.put(jsonObject);
} catch (JSONException e) {
e.printStackTrace();
}
}
// 将 JSON 数组转换为字符串
String jsonString = jsonArray.toString();
// 发送 POST 请求
try {
URL url = new URL("http://example.com/api/");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/json");
connection.setDoOutput(true);
OutputStreamWriter writer = new OutputStreamWriter(connection.getOutputStream());
writer.write(jsonString);
writer.flush();
writer.close();
// 处理服务器返回的响应
// ...
} catch (IOException e) {
e.printStackTrace();
}
```
注意:以上代码仅作为示例,实际情况中你需要根据具体的数据库结构和接口要求来调整代码。另外,为了避免在主线程中执行数据库查询和网络请求,你可能需要使用异步任务或其他线程管理技术。
相关推荐
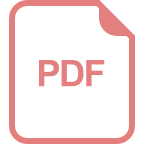
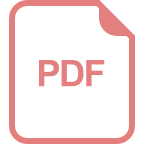














