tcp/udp/icmp校验和计算c代码
时间: 2023-05-17 19:02:28 浏览: 68
以下是一个计算TCP/UDP/ICMP校验和的C代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
uint16_t checksum(uint16_t *buf, int len) {
uint32_t sum = 0;
while (len > 1) {
sum += *buf++;
len -= 2;
}
if (len == 1) {
sum += *(uint8_t*)buf;
}
while (sum >> 16) {
sum = (sum & 0xffff) + (sum >> 16);
}
return ~sum;
}
int main() {
char data[] = "hello world";
int len = strlen(data);
uint16_t *buf = (uint16_t*)data;
uint16_t cksum = checksum(buf, len);
printf("Checksum: 0x%04x\n", cksum);
return 0;
}
```
这个代码示例可以计算任意数据的校验和,包括TCP、UDP和ICMP协议中的数据。
相关问题
windowstcp/udp/icmp校验和计算c代码
以下是计算TCP/UDP/ICMP校验和的C代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
uint16_t checksum(uint16_t *buf, int len) {
uint32_t sum = 0;
while (len > 1) {
sum += *buf++;
len -= 2;
}
if (len == 1) {
sum += *(uint8_t*)buf;
}
while (sum >> 16) {
sum = (sum & 0xffff) + (sum >> 16);
}
return (uint16_t)~sum;
}
int main() {
char data[] = "hello world";
int len = strlen(data);
uint16_t *buf = (uint16_t*)data;
uint16_t result = checksum(buf, len);
printf("Checksum: 0x%x\n", result);
return 0;
}
```
这段代码可以计算任意数据的校验和,只需要将数据转换成16位的字节序列,然后调用checksum函数即可。
windowsTCP/UDP/ICMP校验和计算c代码
以下是计算TCP/UDP/ICMP校验和的C代码:
unsigned short checksum(unsigned short *buf, int nwords)
{
unsigned long sum;
for(sum=0; nwords>0; nwords--)
sum += *buf++;
sum = (sum >> 16) + (sum &0xffff);
sum += (sum >> 16);
return (unsigned short)(~sum);
}
该函数接受一个指向数据缓冲区的指针和缓冲区中的字数。它使用标准的Internet校验和算法来计算校验和,并返回结果。
相关推荐
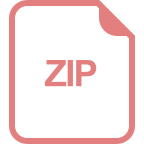












