帮我写一个uni-app项目H5端get请求的功能
时间: 2024-06-09 17:08:04 浏览: 108
好的,以下是一个简单的uni-app项目H5端get请求的代码示例:
1. 在需要发送get请求的页面中,先引入uni-request模块:
```
import uniRequest from 'uni-request';
```
2. 在发送请求的方法中,使用uniRequest.get()方法发送get请求:
```
export default {
methods: {
sendGetRequest() {
uniRequest.get('https://www.example.com/api', {
params: {
id: 123
}
}).then(response => {
console.log(response.data);
}).catch(error => {
console.log(error);
});
}
}
}
```
3. 在get请求的URL中,可以使用params参数传递需要的参数,如上面的例子中的id参数。
4. 请求成功的数据会在response.data中返回,请求失败的错误信息会在error中返回。
希望这个示例能够帮到你。
相关问题
uni-app h5端调用摄像头
在H5端调用摄像头,可以使用HTML5的WebRTC技术,通过getUserMedia API来获取用户媒体设备,包括摄像头、麦克风等。
在uni-app中,你可以使用uni-app提供的uni-app-plus插件来实现在H5端调用摄像头的功能。
首先,在uni-app项目中,需要安装uni-app-plus插件。可以在项目根目录下执行以下命令进行安装:
```
npm install @dcloudio/uni-app-plus --save
```
安装完成后,在需要使用摄像头的页面中,引入plus对象,并调用plus.camera.getCamera()方法来获取摄像头的实例:
```javascript
import uni from 'uni-app-plus';
const plus = uni.requireNativePlugin('plus');
const camera = plus.camera.getCamera();
```
获取到摄像头实例后,可以使用startPreview()方法来启动摄像头预览:
```javascript
camera.startPreview({
index: 0, // 指定摄像头的索引,0为后置摄像头,1为前置摄像头
resolution: 'high', // 指定摄像头分辨率
format: 'jpg', // 指定预览图片的格式
success: function() {
console.log('启动摄像头成功');
},
fail: function(err) {
console.error('启动摄像头失败:' + err.message);
}
});
```
启动摄像头预览后,可以使用takePicture()方法来拍照:
```javascript
camera.takePicture({
quality: 80, // 拍照图片质量
success: function(image) {
console.log('拍照成功,图片地址为:' + image);
},
fail: function(err) {
console.error('拍照失败:' + err.message);
}
});
```
拍照成功后,可以通过返回的image参数获取到拍摄的照片地址。
需要注意的是,使用WebRTC技术获取用户媒体设备需要用户授权,因此在调用摄像头前,需要先请求用户授权。可以使用uni-app提供的uni.authorize()方法来请求用户授权:
```javascript
uni.authorize({
scope: 'scope.camera',
success: function() {
console.log('用户授权成功');
// 调用摄像头相关方法
},
fail: function(err) {
console.error('用户授权失败:' + err.message);
}
});
```
以上就是在uni-app中在H5端调用摄像头的基本流程。
uni-app实现h5跳转app(ios和andiro)指定页面, h5实现功能的完整代码
以下是一个完整的示例代码,包括H5页面中的代码和APP中的代码:
H5页面中的代码:
```
<template>
<div>
<button @click="jumpToApp">跳转到APP</button>
</div>
</template>
<script>
export default {
methods: {
jumpToApp() {
var schemeUrl = "myapp://page?id=123"; // 这里的URL需要根据实际情况进行修改
window.location.href = schemeUrl;
}
}
}
</script>
```
APP中的代码:
```
// iOS
// 在Info.plist文件中添加以下代码
<key>LSApplicationQueriesSchemes</key>
<array>
<string>myapp</string> // 这里的myapp需要和H5中的schemeUrl保持一致
</array>
// 在AppDelegate.m中添加以下代码
- (BOOL)application:(UIApplication *)application
openURL:(NSURL *)url
options:(NSDictionary<UIApplicationOpenURLOptionsKey,id> *)options {
NSString *scheme = [url scheme];
if ([scheme isEqualToString:@"myapp"]) {
NSString *host = [url host];
NSString *path = [url path];
if ([host isEqualToString:@"page"] && [path isEqualToString:@"/"]) {
NSString *query = [url query];
// 根据query参数来获取ID并跳转到对应页面
...
return YES;
}
}
return NO;
}
// Android
// 在AndroidManifest.xml中添加以下代码
<activity
android:name=".MainActivity"
android:launchMode="singleTask"
android:screenOrientation="portrait">
<intent-filter>
<action android:name="android.intent.action.VIEW"/>
<category android:name="android.intent.category.DEFAULT"/>
<category android:name="android.intent.category.BROWSABLE"/>
<data android:scheme="myapp"/> // 这里的myapp需要和H5中的schemeUrl保持一致
</intent-filter>
</activity>
// 在MainActivity中添加以下代码
@Override
protected void onNewIntent(Intent intent) {
super.onNewIntent(intent);
String scheme = intent.getScheme();
if ("myapp".equals(scheme)) {
String host = intent.getData().getHost();
String path = intent.getData().getPath();
if ("page".equals(host) && "/".equals(path)) {
String query = intent.getData().getQuery();
// 根据query参数来获取ID并跳转到对应页面
...
}
}
}
```
以上代码仅仅是一个示例,具体的实现需要根据实际情况进行调整。
阅读全文
相关推荐
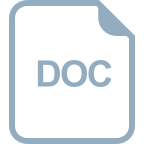
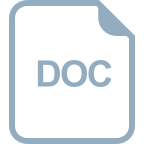
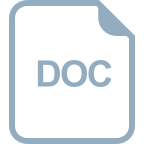
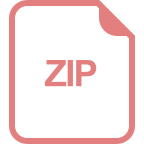
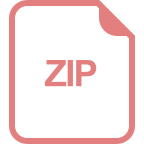



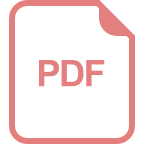
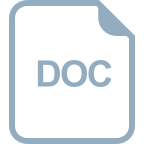
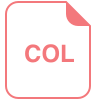





