帮我写一个用esp8266和1306显示B站粉丝的代码
时间: 2024-02-11 15:09:08 浏览: 176
好的,以下是用 ESP8266 和 1306 显示 B 站粉丝数量的代码。
首先,您需要在 B 站开发者平台上创建一个应用程序,并获取到您的应用程序的 App Key 和 App Secret。然后,您需要使用这些凭据来获取 B 站粉丝数量的 API 接口。
您可以使用以下代码来获取 B 站粉丝数量:
```c
#include <ESP8266WiFi.h>
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128
#define SCREEN_HEIGHT 32
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1);
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
const char* host = "api.bilibili.com";
const char* endpoint = "/x/relation/stat?vmid=your_vmid&jsonp=jsonp";
const int httpsPort = 443;
void setup() {
Serial.begin(9600);
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
Wire.begin(4, 5);
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
display.clearDisplay();
display.display();
}
void loop() {
WiFiClientSecure client;
Serial.print("Connecting to ");
Serial.println(host);
if (!client.connect(host, httpsPort)) {
Serial.println("Connection failed");
return;
}
String requestUrl = String("GET ") + endpoint + " HTTP/1.1\r\n" +
"Host: " + host + "\r\n" +
"User-Agent: BuildFailureDetectorESP8266\r\n" +
"Connection: close\r\n\r\n";
Serial.print("Requesting URL: ");
Serial.println(endpoint);
client.print(requestUrl);
while (client.connected()) {
String line = client.readStringUntil('\n');
if (line == "\r") {
break;
}
}
String response = client.readString();
client.stop();
Serial.println("Response:");
Serial.println(response);
int start = response.indexOf("follower\":") + 10;
int end = response.indexOf(",", start);
String followerCountString = response.substring(start, end);
int followerCount = followerCountString.toInt();
Serial.print("B站粉丝数量: ");
Serial.println(followerCount);
display.clearDisplay();
display.setTextSize(1);
display.setTextColor(SSD1306_WHITE);
display.setCursor(0, 0);
display.println("B站粉丝数量:");
display.setTextSize(2);
display.setCursor(0, 10);
display.println(followerCount);
display.display();
delay(60000);
}
```
请将代码中的 `your_SSID` 和 `your_PASSWORD` 替换为您的 WiFi SSID 和密码。将 `your_vmid` 替换为您的 B 站用户 ID。
该代码使用 HTTPS 安全连接到 B 站 API 并提取粉丝数量。然后,它将粉丝数量显示在 1306 OLED 显示屏上。该代码将每分钟更新一次粉丝数量。
希望这可以帮助到您!
阅读全文
相关推荐
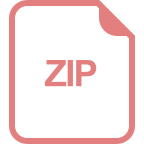



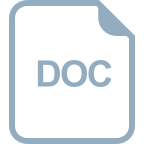
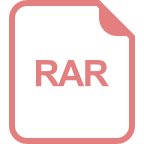
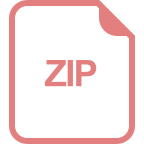
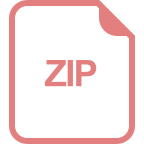
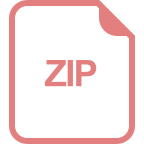
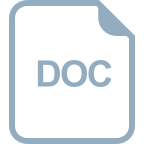
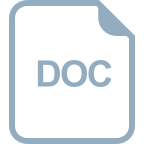
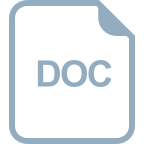
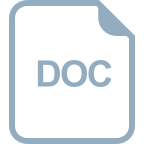
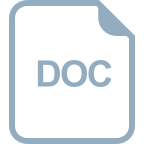
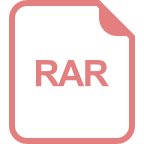