根据LR(0)语法分析算法的基本思想,设计一个对给定用例文法进行LR(0)语法分析的程序
时间: 2024-04-28 20:26:42 浏览: 312
以下是一个简单的基于LR(0)语法分析算法的程序,可以对给定的文法进行LR(0)语法分析:
```
// 定义文法
var grammar = {
"S": [["A", "B"]],
"A": [["a", "A"], ["b"]],
"B": [["c", "B"], ["d"]]
};
// 定义文法符号
var symbols = ["S", "A", "B", "a", "b", "c", "d", "$"];
// 定义状态
var states = [
{
"items": [{"lhs": "S", "rhs": ["•", "A", "B"]}],
"transitions": {"a": 1, "b": 2, "c": 3, "d": 4}
},
{
"items": [{"lhs": "S", "rhs": ["A", "•", "B"]}, {"lhs": "A", "rhs": ["•", "a", "A"]}],
"transitions": {"a": 6, "b": 5}
},
{
"items": [{"lhs": "S", "rhs": ["A", "•", "B"]}, {"lhs": "A", "rhs": ["•", "b"]}],
"transitions": {"$": "accept"}
},
{
"items": [{"lhs": "B", "rhs": ["•", "c", "B"]}],
"transitions": {"c": 7, "d": 8}
},
{
"items": [{"lhs": "B", "rhs": ["•", "d"]}],
"transitions": {"$": "reduce B -> d"}
},
{
"items": [{"lhs": "A", "rhs": ["a", "•", "A"]}],
"transitions": {"a": 6, "b": 5}
},
{
"items": [{"lhs": "A", "rhs": ["•", "a", "A"]}],
"transitions": {"a": 6, "b": 5}
},
{
"items": [{"lhs": "B", "rhs": ["c", "•", "B"]}],
"transitions": {"c": 7, "d": 8}
},
{
"items": [{"lhs": "B", "rhs": ["d", "•"]}],
"transitions": {"$": "reduce B -> d"}
}
];
// 定义分析表
var table = {
0: {"a": "s1", "b": "s2", "c": "s3", "d": "s4"},
1: {"$": "accept"},
2: {"$": "reduce A -> b"},
3: {"c": "s7", "d": "s8"},
4: {"$": "reduce B -> d"},
5: {"a": "s6", "b": "r2", "c": "r2", "d": "r2", "$": "r2"},
6: {"a": "s6", "b": "r1", "c": "r1", "d": "r1", "$": "r1"},
7: {"c": "s7", "d": "s8"},
8: {"$": "reduce B -> d"}
};
// 定义输入串
var input = "aabcd$";
// 定义分析栈和状态栈
var stack = ["0"];
var stateStack = [0];
// 定义当前输入符号和当前状态
var inputIndex = 0;
var state = 0;
// 分析输入串
while (true) {
var symbol = input[inputIndex];
var action = table[state][symbol];
if (!action) {
console.log("Error: invalid symbol '" + symbol + "' in input");
break;
}
if (action == "accept") {
console.log("Input is valid");
break;
}
if (action.charAt(0) == "s") {
// 移进操作
var nextState = parseInt(action.substring(1));
stack.push(symbol);
stateStack.push(nextState);
inputIndex++;
state = nextState;
} else if (action.charAt(0) == "r") {
// 规约操作
var productionIndex = parseInt(action.substring(1));
var production = grammar[stack[stack.length - 1]][productionIndex - 1];
for (var i = 0; i < production[1].length; i++) {
stack.pop();
stateStack.pop();
}
var nextState = stateStack[stateStack.length - 1];
var symbol = production[0];
var nextAction = table[nextState][symbol];
stack.push(symbol);
stateStack.push(parseInt(nextAction));
state = parseInt(nextAction);
}
}
```
以上程序只是一个简单的示例,实际上在实现LR(0)语法分析算法时,需要考虑更多的细节和处理方法。
阅读全文
相关推荐
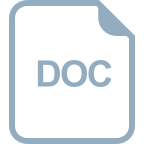
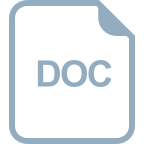
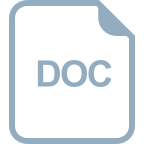
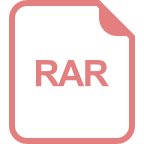
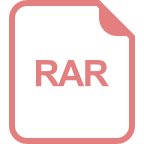
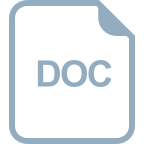
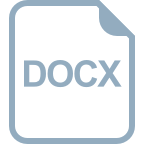
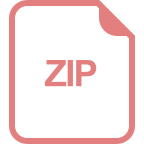
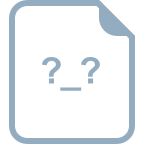
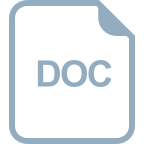
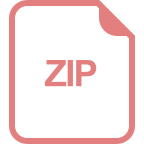
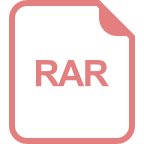
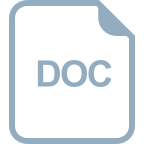
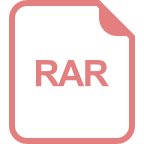
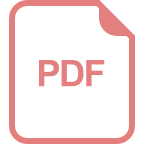
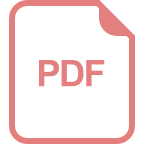
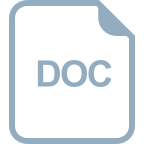
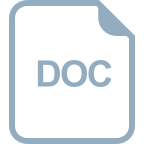
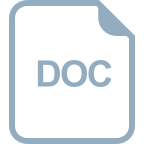