csharp写一个读写ini文件的接口
时间: 2023-06-03 15:01:44 浏览: 188
好的,我可以回答这个问题。你可以使用C#的System.Configuration命名空间中的ConfigurationManager类来读写INI文件。你需要使用AppSetting来读取INI文件中的配置数据,使用ConnectionStrings来读取INI文件中的连接字符串。你也可以使用IniFile类来读取并编写INI文件。
相关问题
用csharp写一个读写ini文件的接口
好的,请稍等,我为您查找相关资料。
关于读写INI文件的接口,您可以使用C#的System.Configuration.ConfigurationManager类来实现。具体步骤如下:
1. 引用System.Configuration命名空间
2. 使用System.Configuration.ConfigurationManager.OpenExeConfiguration方法打开INI文件或者使用ConfigurationManager.AppSettings读取INI文件
3. 使用System.Configuration.Configuration类的Sections和AppSettings属性访问INI文件中的部分和键值对
4. 使用System.Configuration.Configuration类的Save方法保存INI文件
具体代码实现如下:
```csharp
using System.Configuration;
// 读取INI文件
Configuration config = ConfigurationManager.OpenExeConfiguration("file.ini");
string value = config.AppSettings.Settings["key"].Value;
// 写入INI文件
config.AppSettings.Settings["key"].Value = value;
config.Save(ConfigurationSaveMode.Modified);
```
希望这个回答可以帮助您解决问题,如有其他问题,不要犹豫在向我提出。
csharp 创建一个ini文件并读写节点
要创建和读写 INI 文件,可以使用 .NET Framework 中的 System.IO 和 System.Collections 命名空间。以下是一个示例代码,用于创建和读取 INI 文件:
创建 INI 文件:
```csharp
using System.IO;
// 定义 INI 文件路径
string path = @"C:\test.ini";
// 定义节点名称和值
string sectionName = "Section1";
string keyName = "Key1";
string keyValue = "Value1";
// 创建 INI 文件并写入节点
StreamWriter sw = new StreamWriter(path);
sw.WriteLine("[" + sectionName + "]");
sw.WriteLine(keyName + "=" + keyValue);
sw.Close();
```
读取 INI 文件:
```csharp
using System.IO;
using System.Collections;
// 定义 INI 文件路径
string path = @"C:\test.ini";
// 读取 INI 文件并解析节点
StreamReader sr = new StreamReader(path);
Hashtable sections = new Hashtable();
string section = null;
while (!sr.EndOfStream)
{
string line = sr.ReadLine().Trim();
if (line.StartsWith("[") && line.EndsWith("]"))
{
section = line.Substring(1, line.Length - 2);
sections.Add(section, new Hashtable());
}
else if (section != null && line.Contains("="))
{
string[] parts = line.Split(new char[] { '=' }, 2);
string key = parts[0].Trim();
string value = parts[1].Trim();
((Hashtable)sections[section])[key] = value;
}
}
sr.Close();
// 获取节点值
string sectionName = "Section1";
string keyName = "Key1";
string keyValue = (string)((Hashtable)sections[sectionName])[keyName];
Console.WriteLine(keyValue);
```
阅读全文
相关推荐




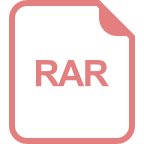
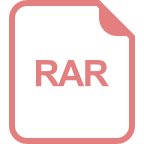

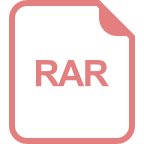
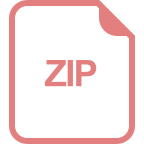



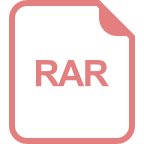
