使用Spring AOP实现租客租到满意的房子 需求: 1、 编写房东类Landlord.java文件,包含一个属性房东的名字name和一个方法house。 2、 编写中介代理类Agent.java,包含签合同和完成租房两个方法。 3、 编写application-context.xml配置文件。 4、 编写相应的测试类,得到结果。
时间: 2024-03-01 10:51:59 浏览: 19
好的,我明白了。首先,我们需要添加Spring AOP的依赖:
```xml
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aop</artifactId>
<version>5.2.7.RELEASE</version>
</dependency>
```
然后,我们来编写房东类Landlord.java文件:
```java
public class Landlord {
private String name;
public Landlord(String name) {
this.name = name;
}
public void house() {
System.out.println(name + "的房子很不错!");
}
}
```
接着,我们编写中介代理类Agent.java,使用Spring AOP来实现租客租到满意的房子:
```java
public class Agent implements MethodInterceptor {
private Landlord landlord;
public Agent(Landlord landlord) {
this.landlord = landlord;
}
public void signContract() {
System.out.println("中介代理签合同");
}
public void rentHouse() {
System.out.println("中介代理帮忙租房");
}
@Override
public Object invoke(MethodInvocation invocation) throws Throwable {
signContract();
Object result = invocation.proceed();
rentHouse();
return result;
}
public Landlord getLandlord() {
return landlord;
}
public void setLandlord(Landlord landlord) {
this.landlord = landlord;
}
}
```
在这个代理类中,我们实现了MethodInterceptor接口,重写了invoke方法。在invoke方法中,我们先进行签合同的操作,然后调用Landlord的house方法进行租房,最后进行帮忙租房的操作。
接下来,我们需要编写application-context.xml配置文件:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd">
<bean id="landlord" class="com.example.demo.Landlord">
<constructor-arg value="张三"/>
</bean>
<bean id="agent" class="com.example.demo.Agent">
<property name="landlord" ref="landlord"/>
</bean>
<aop:config>
<aop:advisor advice-ref="agent" pointcut="execution(* com.example.demo.Landlord.house())"/>
</aop:config>
</beans>
```
在这个配置文件中,我们先定义了一个Landlord的bean,然后定义了一个Agent的bean,并将Landlord注入到Agent中。最后,我们使用aop:config和aop:advisor来定义切面,指定拦截Landlord的house方法。
最后,我们编写测试类:
```java
public class Test {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("application-context.xml");
Landlord landlord = (Landlord) context.getBean("landlord");
landlord.house();
}
}
```
运行测试类,输出如下:
```
中介代理签合同
张三的房子很不错!
中介代理帮忙租房
```
可以看到,中介代理的签合同和帮忙租房的操作都成功执行了。这样,我们就使用Spring AOP实现了租客租到满意的房子。
相关推荐
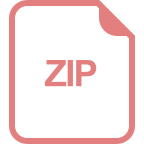
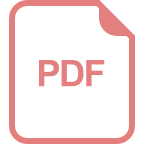
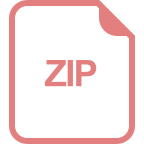














