pos-lssvmpython代码
时间: 2023-10-20 22:05:19 浏览: 130
以下是一个简单的基于 LSSVM 的二分类器的 Python 代码示例:
```python
import numpy as np
from cvxopt import matrix, solvers
class LSSVM:
def __init__(self, kernel='linear', C=1.0, gamma=None):
self.kernel = kernel
self.C = C
self.gamma = gamma
self.alpha = None
self.w0 = None
self.X = None
self.y = None
def fit(self, X, y):
self.X = X
self.y = y
n_samples, n_features = X.shape
if self.gamma is None:
self.gamma = 1.0 / n_features
K = self._kernel_matrix(X, self.X)
P = matrix(np.outer(y, y) * K)
q = matrix(-np.ones(n_samples))
G = matrix(np.vstack((-np.eye(n_samples), np.eye(n_samples))))
h = matrix(np.hstack((np.zeros(n_samples), self.C * np.ones(n_samples))))
A = matrix(y.reshape(1, -1))
b = matrix(np.zeros(1))
solvers.options['show_progress'] = False
sol = solvers.qp(P, q, G, h, A, b)
self.alpha = np.array(sol['x']).flatten()
sv_idxs = self.alpha > 1e-5
self.w0 = np.mean(y[sv_idxs] - np.sum(self.alpha[sv_idxs].reshape(-1, 1) * y[sv_idxs].reshape(-1, 1) * K[sv_idxs, sv_idxs], axis=0))
def predict(self, X):
K = self._kernel_matrix(X, self.X)
y_pred = np.sum(self.alpha.reshape(-1, 1) * self.y.reshape(-1, 1) * K, axis=0) + self.w0
return np.sign(y_pred)
def _kernel_matrix(self, X1, X2):
if self.kernel == 'linear':
return np.dot(X1, X2.T)
elif self.kernel == 'rbf':
sq_dists = np.sum(X1**2, axis=1)[:, np.newaxis] + np.sum(X2**2, axis=1) - 2 * np.dot(X1, X2.T)
return np.exp(-self.gamma * sq_dists)
else:
raise ValueError('Unknown kernel')
```
该代码实现了一个简单的 LSSVM 二分类器,支持线性核和 RBF 核。内部使用了 CVXOPT 求解二次规划问题。`fit()` 方法用于训练模型,`predict()` 方法用于预测新样本的类别。
阅读全文
相关推荐
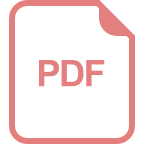
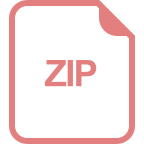
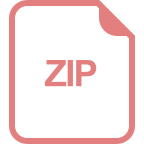
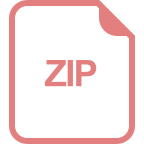
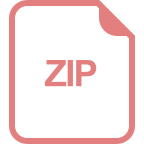
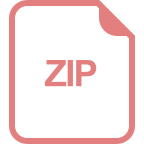
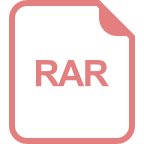
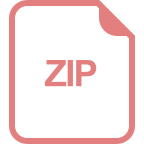
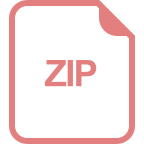
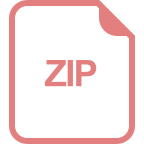
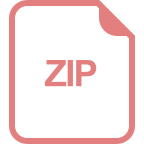
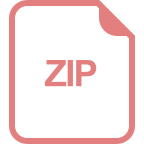
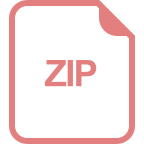
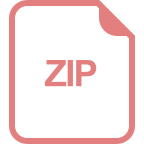
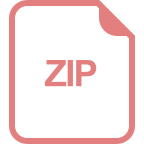
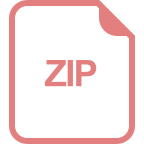