c语言读取txt文件数据
时间: 2023-08-29 10:08:14 浏览: 76
你可以使用C语言的标准库函数fopen、fscanf、fclose来读取txt文件数据。具体步骤如下:
1. 使用fopen函数打开txt文件,例如:
```
FILE *fp;
fp = fopen("example.txt", "r");
```
其中,"example.txt"是你要读取的文件名,"r"表示以只读方式打开文件。
2. 使用fscanf函数读取文件中的数据,例如:
```
int num1, num2;
fscanf(fp, "%d %d", &num1, &num2);
```
其中,"%d %d"是指文件中的数据格式为两个整数,&num1和&num2是读取到的数据存储的变量地址。
3. 使用fclose函数关闭文件,例如:
```
fclose(fp);
```
完整的代码示例:
```
#include <stdio.h>
int main() {
FILE *fp;
int num1, num2;
fp = fopen("example.txt", "r");
if(fp == NULL) {
printf("Error opening file.");
return 1;
}
fscanf(fp, "%d %d", &num1, &num2);
printf("Read numbers: %d, %d", num1, num2);
fclose(fp);
return 0;
}
```
注意,在使用fscanf函数时,要确保文件中的数据格式与读取格式一致,否则会导致读取错误。
阅读全文
相关推荐










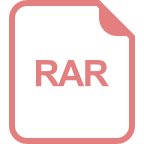

