spring boot controller入参body统一解密的实现方式有哪些
时间: 2024-01-29 12:03:47 浏览: 157
在Spring Boot中,可以通过自定义拦截器或注解来实现Controller入参Body统一解密的功能。下面是两种实现方式:
1. 自定义拦截器
自定义一个拦截器,通过拦截请求,在进入Controller方法之前对RequestBody进行解密,然后再将解密后的参数传递给Controller方法处理。
示例代码:
```java
public class DecryptRequestBodyInterceptor implements HandlerInterceptor {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
if (handler instanceof HandlerMethod) {
HandlerMethod handlerMethod = (HandlerMethod) handler;
Method method = handlerMethod.getMethod();
if (method.isAnnotationPresent(DecryptRequestBody.class)) {
String requestBody = request.getReader().lines().collect(Collectors.joining(System.lineSeparator()));
String decryptedRequestBody = decrypt(requestBody); // 解密RequestBody
request.setAttribute("decryptedRequestBody", decryptedRequestBody); // 将解密后的参数放入Request中
}
}
return true;
}
}
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface DecryptRequestBody {
}
@RestController
public class ExampleController {
@PostMapping("/example")
@DecryptRequestBody // 标注需要进行解密的方法
public String example(@RequestBody String requestBody, HttpServletRequest request) {
String decryptedRequestBody = (String) request.getAttribute("decryptedRequestBody"); // 从Request中获取解密后的参数
return "Decrypted RequestBody: " + decryptedRequestBody;
}
}
```
2. 自定义注解
自定义一个注解,标注需要进行解密的Controller方法,在进入Controller方法之前对注解标注的RequestBody进行解密,然后再将解密后的参数传递给Controller方法处理。
示例代码:
```java
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface DecryptRequestBody {
}
@Aspect
@Component
public class DecryptRequestBodyAspect {
@Autowired
private ObjectMapper objectMapper;
@Around("@annotation(cn.example.annotation.DecryptRequestBody)")
public Object decryptRequestBody(ProceedingJoinPoint pjp) throws Throwable {
ServletRequestAttributes requestAttributes = (ServletRequestAttributes) RequestContextHolder.getRequestAttributes();
HttpServletRequest request = requestAttributes.getRequest();
String requestBody = request.getReader().lines().collect(Collectors.joining(System.lineSeparator()));
String decryptedRequestBody = decrypt(requestBody); // 解密RequestBody
Object[] args = pjp.getArgs();
for (int i = 0; i < args.length; i++) {
if (args[i] instanceof String && AnnotationUtils.findAnnotation(pjp.getSignature().getDeclaringType().getDeclaredMethod(pjp.getSignature().getName(), String.class).getParameterAnnotations()[i], RequestBody.class) != null) {
args[i] = objectMapper.readValue(decryptedRequestBody, pjp.getSignature().getParameterTypes()[i]); // 将解密后的参数转换为Controller方法参数类型
}
}
return pjp.proceed(args);
}
}
@RestController
public class ExampleController {
@PostMapping("/example")
@DecryptRequestBody // 标注需要进行解密的方法
public String example(@RequestBody ExampleRequest request) {
return "Decrypted RequestBody: " + request.getContent();
}
}
public class ExampleRequest {
private String content;
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
}
```
以上两种实现方式都可以实现Controller入参Body统一解密的功能,具体选择哪种实现方式取决于实际需求。
阅读全文
相关推荐
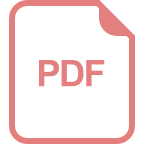
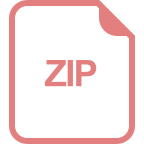
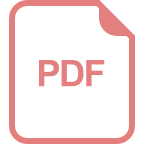
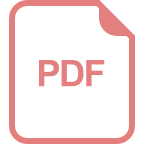
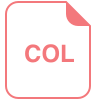
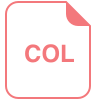
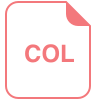
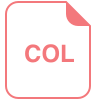
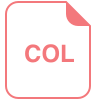
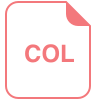
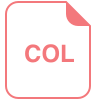
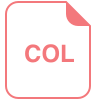
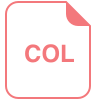
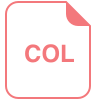
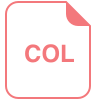
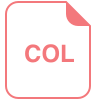
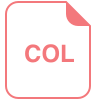
