利用beautifulsoup如何获取多个标签a下的多个href
时间: 2024-06-10 14:03:46 浏览: 119
?
你可以使用beautifulsoup的find_all方法来获取多个标签a,然后使用for循环遍历每个a标签,再使用.get('href')方法获取每个a标签下的href属性值。以下是一个示例代码:
```
from bs4 import BeautifulSoup
import requests
# 构建网页请求
url = 'http://example.com'
response = requests.get(url)
# 解析网页内容
soup = BeautifulSoup(response.text, 'html.parser')
# 获取所有的a标签
a_tags = soup.find_all('a')
for a in a_tags:
href = a.get('href')
if href is not None:
print(href)
```
这段代码将会输出所有a标签下的href属性值。
相关问题
利用beautifulsoup如何获取标签div下的a标签
利用BeautifulSoup可以通过以下代码获取标签div下的a标签:
```python
from bs4 import BeautifulSoup
html = '<div><a href="https://www.example.com">Link text</a></div>'
soup = BeautifulSoup(html, 'html.parser')
div_tag = soup.find('div')
a_tag = div_tag.find('a')
print(a_tag)
```
其中,`find()` 方法可以用来查找指定的标签,而 `div_tag.find('a')` 语句则是在 div 标签下查找 a 标签。如果页面中有多个 div 标签,可以使用 `find_all('div')` 方法获取所有的 div 标签,之后再遍历所有的 div 标签,并查找其下的 a 标签。
BeautifulSoup如何遍历a标签下b标签内容
要遍历a标签下的b标签内容,您可以使用BeautifulSoup的find_all()方法来查找所有a标签,然后使用for循环遍历每个a标签,并使用find_all()方法再次查找a标签下的所有b标签。以下是一个示例代码:
```python
from bs4 import BeautifulSoup
# 假设html是包含a标签和b标签的HTML代码
html = """
<a href="https://example.com">
<b>Link 1</b>
</a>
<a href="https://example.com">
<b>Link 2</b>
</a>
<a href="https://example.com">
<b>Link 3</b>
</a>
"""
# 使用BeautifulSoup解析HTML
soup = BeautifulSoup(html, 'html.parser')
# 查找所有a标签
a_tags = soup.find_all('a')
# 遍历每个a标签
for a_tag in a_tags:
# 查找a标签下的所有b标签
b_tags = a_tag.find_all('b')
# 遍历每个b标签并打印内容
for b_tag in b_tags:
print(b_tag.text)
```
运行上述代码后,将输出以下内容:
```
Link 1
Link 2
Link 3
```
这样就可以遍历a标签下的b标签内容了。请注意,此示例假设HTML代码中只有一个a标签,如果有多个a标签,将会遍历每个a标签下的子标签。
阅读全文
相关推荐
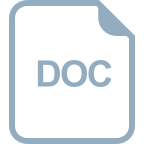
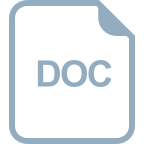
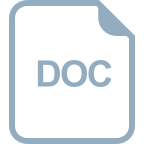
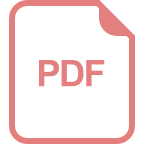
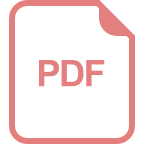
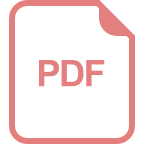
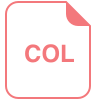
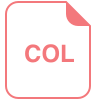
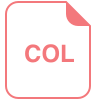







