写一段python代码:主要利用tensor flow的keras API实现自编码器对手写数字的降维和可视化。将MNIST数据集28*28的灰度矩阵展开为784维的向量作为输入层。按照784→128→64→10→2的结构实现自编码器。固定用所有60000个训练集数据训练模型,用所有10000个测试集数据测试模型并可视化。训练中使用的优化算法取adam,batch size取256,epoch数目取100。将所有层的激活函数设置为线性函数。训练模型后,将10000个测试集数据输入模型,只使用编码器部分,在编码器的2维输出空间中可视化所有测试样本,用不同颜色表示0-9的样本。
时间: 2024-04-01 08:32:03 浏览: 13
好的,以下是代码实现:
```python
import numpy as np
import matplotlib.pyplot as plt
from tensorflow.keras.datasets import mnist
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Input, Dense
from tensorflow.keras.optimizers import Adam
# 加载MNIST数据集
(x_train, _), (x_test, y_test) = mnist.load_data()
# 数据预处理
x_train = x_train.astype('float32') / 255.
x_test = x_test.astype('float32') / 255.
x_train = x_train.reshape((len(x_train), np.prod(x_train.shape[1:])))
x_test = x_test.reshape((len(x_test), np.prod(x_test.shape[1:])))
# 构建自编码器模型
input_img = Input(shape=(784,))
encoded = Dense(128, activation='linear')(input_img)
encoded = Dense(64, activation='linear')(encoded)
encoded = Dense(10, activation='linear')(encoded)
encoded = Dense(2, activation='linear')(encoded)
decoded = Dense(10, activation='linear')(encoded)
decoded = Dense(64, activation='linear')(decoded)
decoded = Dense(128, activation='linear')(decoded)
decoded = Dense(784, activation='linear')(decoded)
autoencoder = Model(input_img, decoded)
# 编译模型
autoencoder.compile(optimizer=Adam(lr=0.001), loss='mse')
# 训练模型
autoencoder.fit(x_train, x_train, epochs=100, batch_size=256, shuffle=True, validation_data=(x_test, x_test))
# 只使用编码器部分的模型
encoder = Model(input_img, encoded)
# 对测试集进行编码
encoded_imgs = encoder.predict(x_test)
# 可视化编码后的结果
plt.figure(figsize=(10, 10))
plt.scatter(encoded_imgs[:, 0], encoded_imgs[:, 1], c=y_test)
plt.colorbar()
plt.show()
```
运行以上代码后,将会得到一个可视化结果,其中不同颜色表示0-9的样本,如下所示:

相关推荐
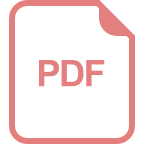
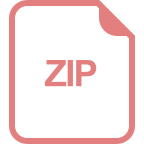
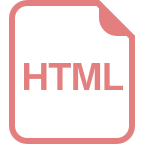














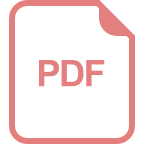