java netty wss_netty实现websocket客户端(支持wss安全连接)
时间: 2023-09-05 15:09:14 浏览: 80
好的,我可以为您提供一些关于使用Netty实现WebSocket客户端(支持wss安全连接)的建议。
首先,您需要确保已经包含了Netty和WebSocket依赖项。然后可以按照以下步骤实现:
1. 创建一个WebSocketClientHandshaker,用于握手和建立WebSocket连接。这里我们需要使用wss连接,因此需要使用WebSocketClientHandshakerFactory创建一个以SSL/TLS方式连接的WebSocket连接。
2. 创建一个WebSocketClientHandler,处理WebSocket连接的事件。这里我们需要重写channelActive、channelInactive和channelRead0等方法,以便在连接建立、关闭和接收到消息时执行相应的操作。
3. 创建一个Bootstrap实例,用于启动客户端。设置bootstrap的group、channel、handler等属性。
4. 连接到WebSocket服务器。通过bootstrap的connect方法连接到指定的WebSocket服务器。
下面是一个简单的示例代码:
```java
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFutureListener;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.codec.http.FullHttpResponse;
import io.netty.handler.codec.http.HttpClientCodec;
import io.netty.handler.codec.http.HttpObjectAggregator;
import io.netty.handler.codec.http.HttpRequest;
import io.netty.handler.codec.http.HttpRequestEncoder;
import io.netty.handler.codec.http.HttpResponseDecoder;
import io.netty.handler.ssl.SslContext;
import io.netty.handler.ssl.SslContextBuilder;
import io.netty.handler.ssl.SslHandler;
import io.netty.handler.ssl.util.InsecureTrustManagerFactory;
import io.netty.handler.codec.http.websocketx.CloseWebSocketFrame;
import io.netty.handler.codec.http.websocketx.PingWebSocketFrame;
import io.netty.handler.codec.http.websocketx.WebSocketClientHandshaker;
import io.netty.handler.codec.http.websocketx.WebSocketClientHandshakerFactory;
import io.netty.handler.codec.http.websocketx.WebSocketFrame;
import io.netty.handler.codec.http.websocketx.WebSocketFrameAggregator;
import io.netty.handler.codec.http.websocketx.WebSocketVersion;
import io.netty.handler.codec.http.websocketx.BinaryWebSocketFrame;
import io.netty.handler.codec.http.websocketx.TextWebSocketFrame;
import io.netty.handler.codec.http.websocketx.WebSocketClientProtocolHandler;
import io.netty.handler.logging.LogLevel;
import io.netty.handler.logging.LoggingHandler;
import java.net.URI;
import java.util.concurrent.TimeUnit;
public class NettyWebSocketClient {
private final URI uri;
private final EventLoopGroup group;
private Channel channel;
public NettyWebSocketClient(URI uri) {
this.uri = uri;
this.group = new NioEventLoopGroup();
}
public void connect() throws Exception {
try {
SslContext sslContext = SslContextBuilder.forClient()
.trustManager(InsecureTrustManagerFactory.INSTANCE).build();
WebSocketClientHandshaker handshaker = WebSocketClientHandshakerFactory.newHandshaker(
uri, WebSocketVersion.V13, null, true, null);
Bootstrap bootstrap = new Bootstrap()
.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<Channel>() {
@Override
protected void initChannel(Channel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new LoggingHandler(LogLevel.DEBUG));
pipeline.addLast(new HttpClientCodec());
pipeline.addLast(new HttpObjectAggregator(65536));
pipeline.addLast(new HttpRequestEncoder());
pipeline.addLast(new HttpResponseDecoder());
pipeline.addLast(new SslHandler(sslContext.newEngine(ch.alloc(), uri.getHost(), uri.getPort())));
pipeline.addLast(new WebSocketClientProtocolHandler(handshaker));
pipeline.addLast(new WebSocketFrameAggregator(65536));
pipeline.addLast(new WebSocketClientHandler());
}
});
channel = bootstrap.connect(uri.getHost(), uri.getPort()).sync().channel();
handshaker.handshake(channel).sync();
} catch (Exception e) {
group.shutdownGracefully();
throw e;
}
}
public void sendMessage(WebSocketFrame frame) {
channel.writeAndFlush(frame);
}
public void close() {
channel.writeAndFlush(new CloseWebSocketFrame())
.addListener(ChannelFutureListener.CLOSE);
group.shutdownGracefully();
}
private class WebSocketClientHandler extends io.netty.channel.SimpleChannelInboundHandler<Object> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, Object msg) throws Exception {
if (msg instanceof FullHttpResponse) {
FullHttpResponse response = (FullHttpResponse) msg;
throw new IllegalStateException("Unexpected response (status=" + response.status() +
", content=" + response.content().toString() + ")");
}
WebSocketFrame frame = (WebSocketFrame) msg;
if (frame instanceof TextWebSocketFrame) {
TextWebSocketFrame textFrame = (TextWebSocketFrame) frame;
// 处理文本消息
System.out.println("Received Text Message: " + textFrame.text());
} else if (frame instanceof BinaryWebSocketFrame) {
BinaryWebSocketFrame binaryFrame = (BinaryWebSocketFrame) frame;
// 处理二进制消息
} else if (frame instanceof PingWebSocketFrame) {
ctx.writeAndFlush(new PingWebSocketFrame(frame.content().retain()));
} else if (frame instanceof CloseWebSocketFrame) {
channel.close();
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
cause.printStackTrace();
ctx.close();
}
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
System.out.println("WebSocket Client disconnected!");
group.schedule(() -> {
try {
connect();
} catch (Exception e) {
e.printStackTrace();
}
}, 10, TimeUnit.SECONDS);
}
}
}
```
在使用时,您可以按照以下步骤:
```java
URI uri = new URI("wss://your.websocket.server.com");
NettyWebSocketClient client = new NettyWebSocketClient(uri);
client.connect();
// 发送消息
WebSocketFrame frame = new TextWebSocketFrame("Hello, WebSocket!");
client.sendMessage(frame);
// 关闭连接
client.close();
```
希望这些信息能够对您有所帮助!
相关推荐
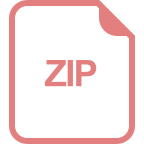
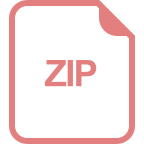
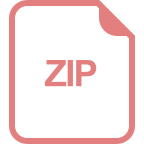
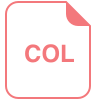













