oid servo_set_angle(int channel, int angle) { int pulse_width = SERVO_MIN + (SERVO_RANGE * angle / 180); pca9685_set_pwm(channel, 0, pulse_width * 4096 / 20000); }什么意思,具体如何使用
时间: 2023-06-17 18:03:42 浏览: 171
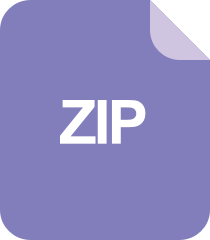
snmpv1.zip_SNMP_SNMP set_snmp get_snmp java_snmp++

这段代码是一个函数,用于控制舵机的角度。其中:
- `channel` 表示要控制的舵机通道号;
- `angle` 表示要设置的舵机角度。
函数的具体实现是先根据 `SERVO_MIN` 和 `SERVO_RANGE` 计算出对应角度下的脉冲宽度,再通过 `pca9685_set_pwm` 函数将脉冲宽度转换成 PWM 信号输出到 PCA9685 芯片上,从而控制舵机转动到指定角度。
使用时,需要先初始化 PCA9685 芯片,并调用 `servo_set_angle` 函数来控制舵机的角度。例如,假设我们要控制第一个通道的舵机转动到 90 度,可以这样写:
```
pca9685_init(); // 初始化 PCA9685 芯片
servo_set_angle(0, 90); // 控制第一个通道的舵机转动到 90 度
```
阅读全文
相关推荐
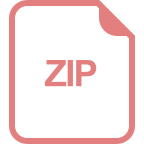
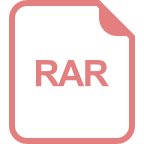

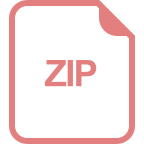














