用java写一个双色球的中奖代码
时间: 2024-03-08 10:48:15 浏览: 175
好的,以下是一个简单的Java程序,用于判断双色球彩票中奖情况:
```java
import java.util.Arrays;
import java.util.Random;
import java.util.Scanner;
public class DoubleColorBall {
public static void main(String[] args) {
int[] redBalls = new int[6]; // 存放6个红球号码的数组
int blueBall; // 存放1个蓝球号码的变量
int[] winningNumbers = generateWinningNumbers(); // 生成中奖号码
System.out.println("中奖号码为:" + Arrays.toString(winningNumbers));
Scanner scanner = new Scanner(System.in);
System.out.println("请输入6个红球号码(1~33之间的整数):");
for (int i = 0; i < redBalls.length; i++) {
redBalls[i] = scanner.nextInt();
}
System.out.println("请输入1个蓝球号码(1~16之间的整数):");
blueBall = scanner.nextInt();
int redBallMatches = countMatches(redBalls, winningNumbers); // 计算红球中奖数
boolean hasBlueBallMatch = blueBall == winningNumbers[6]; // 判断是否中了蓝球
if (redBallMatches == 6 && hasBlueBallMatch) {
System.out.println("恭喜你,中了一等奖!");
} else if (redBallMatches == 6) {
System.out.println("恭喜你,中了二等奖!");
} else if (redBallMatches == 5 && hasBlueBallMatch) {
System.out.println("恭喜你,中了三等奖!");
} else if (redBallMatches == 5 || (redBallMatches == 4 && hasBlueBallMatch)) {
System.out.println("恭喜你,中了四等奖!");
} else if (redBallMatches == 4 || (redBallMatches == 3 && hasBlueBallMatch)) {
System.out.println("恭喜你,中了五等奖!");
} else if (hasBlueBallMatch) {
System.out.println("恭喜你,中了六等奖!");
} else {
System.out.println("很遗憾,你没有中奖!");
}
}
/**
* 生成中奖号码
*/
private static int[] generateWinningNumbers() {
int[] redBalls = new int[6];
Random random = new Random();
for (int i = 0; i < redBalls.length; i++) {
redBalls[i] = random.nextInt(33) + 1;
for (int j = 0; j < i; j++) {
if (redBalls[i] == redBalls[j]) {
i--;
break;
}
}
}
Arrays.sort(redBalls);
int blueBall = random.nextInt(16) + 1;
return Arrays.copyOf(redBalls, 7); // 将红球号码和蓝球号码合并成一个数组返回
}
/**
* 计算两个数组中相同元素的个数
*/
private static int countMatches(int[] arr1, int[] arr2) {
int count = 0;
for (int i = 0; i < arr1.length; i++) {
for (int j = 0; j < arr2.length; j++) {
if (arr1[i] == arr2[j]) {
count++;
break;
}
}
}
return count;
}
}
```
该程序首先生成一个随机的中奖号码,然后提示用户输入6个红球号码和1个蓝球号码,接着计算用户选择的号码与中奖号码的匹配情况,并输出中奖等级。注意,该程序只是一个简单的示例,仅供参考,实际中奖情况需要参考彩票规则来确定。
阅读全文
相关推荐
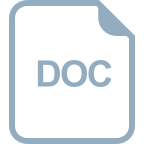
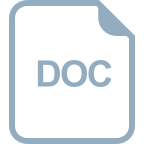
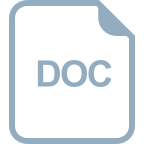











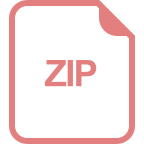
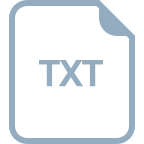