写一个SIFT-SFM算法
时间: 2023-08-02 20:34:27 浏览: 80
SIFT(Scale-Invariant Feature Transform)算法是一种基于尺度空间的特征点检测和描述方法。SFM(Structure-from-Motion)算法是一种基于多张图像的三维重建方法。下面是一个简单的SIFT-SFM算法的实现:
1. 首先,使用OpenCV中的SIFT算法对多张图像进行特征点检测和描述。
```python
import cv2
def detect_and_compute(image):
sift = cv2.xfeatures2d.SIFT_create()
keypoints, descriptors = sift.detectAndCompute(image, None)
return keypoints, descriptors
```
2. 对于每张图像,使用FLANN算法对其特征点进行匹配。
```python
import numpy as np
def match_features(descriptors1, descriptors2):
# 创建FLANN匹配器
flann = cv2.FlannBasedMatcher_create()
# 将第一张图像的描述子和第二张图像的描述子进行匹配
matches = flann.knnMatch(descriptors1, descriptors2, k=2)
# 根据最近邻和次近邻之间的距离比值,筛选出好的匹配点
good_matches = []
for m, n in matches:
if m.distance < 0.7 * n.distance:
good_matches.append(m)
return good_matches
```
3. 对于至少三张匹配的图像,使用OpenCV中的SFM算法进行三维重建。
```python
def reconstruct_3d(matches, keypoints_list, camera_matrices):
# 如果匹配的图像不足3张,则返回None
if len(matches) < 3:
return None
# 构建三维点列表和二维点列表
points3d = []
points2d = []
for match in matches:
img_idx1 = match.queryIdx
img_idx2 = match.trainIdx
kp1 = keypoints_list[img_idx1]
kp2 = keypoints_list[img_idx2]
point3d = cv2.triangulatePoints(camera_matrices[0], camera_matrices[1], kp1.pt, kp2.pt)
points3d.append(point3d)
points2d.append((kp1.pt, kp2.pt))
# 将三维点列表和二维点列表转换成数组
points3d = np.array(points3d)
points2d = np.array(points2d)
# 使用OpenCV中的SFM算法进行三维重建
success, camera_matrix, rotation_vector, translation_vector, point3d = cv2.solvePnPRansac(points3d, points2d, camera_matrices[0], None)
return point3d
```
4. 对于每组匹配的图像,使用RANSAC算法进行三维重建,并将所有的三维点合并在一起。
```python
def reconstruct_all_3d(matches_list, keypoints_list, camera_matrices):
all_points3d = []
for matches in matches_list:
points3d = reconstruct_3d(matches, keypoints_list, camera_matrices)
if points3d is not None:
all_points3d.extend(points3d)
all_points3d = np.array(all_points3d)
return all_points3d
```
以上是一个简单的SIFT-SFM算法的实现,仅供参考。实际应用中,需要根据具体的数据和任务进行调整和优化。
相关推荐
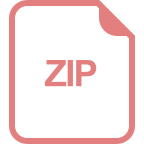
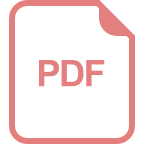
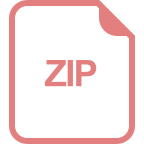















