def _save_file(self, fileName): writer = QImageWriter(fileName) native_filename = QDir.toNativeSeparators(fileName) if not writer.write(self._image): error = writer.errorString() message = f"Cannot write {native_filename}: {error}" QMessageBox.information(self, QGuiApplication.applicationDisplayName(), message) return False self.statusBar().showMessage(f'Wrote "{native_filename}"') return True
时间: 2024-02-10 16:35:27 浏览: 84
这段代码定义了一个 `_save_file()` 方法,用于将当前图像保存到指定的文件中。
该方法接受一个文件名作为参数,并使用 `QImageWriter` 类创建一个 `QImageWriter` 对象,用于将图像写入文件。然后,该方法使用 `QDir.toNativeSeparators()` 方法将文件名转换为本地文件名格式,以便在不同操作系统上都可以正常工作。
接着,该方法使用 `QImageWriter.write()` 方法将图像写入文件。如果写入失败,则使用 `QMessageBox.information()` 方法显示一个消息框,提示用户写入文件时出现了错误,并返回 False。
如果写入成功,则使用 `self.statusBar().showMessage()` 方法在状态栏中显示一个消息,提示用户成功写入文件,并返回 True。
总的来说,这个方法用于将当前图像保存到指定的文件中,并提供了错误处理和状态提示功能。
相关问题
def load_file(self, fileName): reader = QImageReader(fileName) reader.setAutoTransform(True) new_image = reader.read() native_filename = QDir.toNativeSeparators(fileName) if new_image.isNull(): error = reader.errorString() QMessageBox.information(self, QGuiApplication.applicationDisplayName(), f"Cannot load {native_filename}: {error}") return False self._set_image(new_image) self.setWindowFilePath(fileName) w = self._image.width() h = self._image.height() d = self._image.depth() color_space = self._image.colorSpace() description = color_space.description() if color_space.isValid() else 'unknown' message = f'Opened "{native_filename}", {w}x{h}, Depth: {d} ({description})' self.statusBar().showMessage(message) return True
这段代码定义了一个名为 load_file() 的方法,用于加载指定的图像文件并将其显示在主窗口中。
首先,该方法使用 QImageReader 类读取指定的图像文件。setAutoTransform(True) 方法用于启用自动转换功能,可以自动旋转图像以正确显示其方向。
如果读取失败,则会显示一个消息框提示用户。否则,该方法调用 _set_image() 方法将读取的图像设置为当前显示的图像,并将主窗口的标题设置为打开的文件名。
接着,该方法获取图像的宽度、高度、位深、颜色空间等信息,并在状态栏中显示这些信息。
最后,该方法返回 True 表示加载成功。
if self._first_file_dialog: self._first_file_dialog = False locations = QStandardPaths.standardLocations(QStandardPaths.PicturesLocation) directory = locations[-1] if locations else QDir.currentPath() dialog.setDirectory(directory) mime_types = [m.data().decode('utf-8') for m in QImageWriter.supportedMimeTypes()] mime_types.sort() dialog.setMimeTypeFilters(mime_types) dialog.selectMimeTypeFilter("image/jpeg") dialog.setAcceptMode(acceptMode) if acceptMode == QFileDialog.AcceptSave: dialog.setDefaultSuffix("jpg")
这段代码是 `QFileDialog` 对话框的一部分,它用于设置文件对话框的属性和过滤器。
首先,我们检查 `_first_file_dialog` 变量的值。如果它为 True,则说明这是第一次打开文件对话框,我们需要设置对话框的默认目录为系统中的图片目录。我们使用 `QStandardPaths.standardLocations()` 方法获取系统中的图片目录,然后将最后一个目录设置为默认目录。如果系统中没有设置图片目录,则将当前目录设置为默认目录。最后,我们将 `_first_file_dialog` 变量的值设置为 False,以便在后续打开文件对话框时不再设置默认目录。
然后,我们使用 `QImageWriter.supportedMimeTypes()` 方法获取支持的 MIME 类型,并将其转换为字符串列表 `mime_types`,并按字母顺序排序。然后,我们使用 `setMimeTypeFilters()` 方法将 MIME 类型过滤器设置为 `mime_types`。这样,用户只能选择支持的 MIME 类型的文件。
接下来,我们使用 `selectMimeTypeFilter()` 方法将默认的 MIME 类型过滤器设置为 "image/jpeg"。这样,当用户打开文件对话框时,默认选择 JPEG 格式的文件。
最后,我们使用 `setDefaultSuffix()` 方法将默认后缀设置为 "jpg"。这样,在用户选择保存文件时,程序将使用 ".jpg" 作为文件名的扩展名。需要注意的是,`setDefaultSuffix()` 方法只有在文件对话框的 acceptMode 属性设置为 `QFileDialog.AcceptSave` 时才起作用。
阅读全文
相关推荐
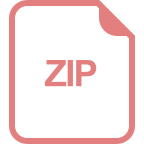
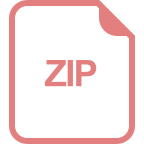
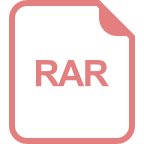













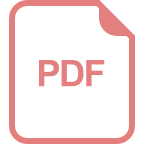