c#根据窗口句柄获取窗口截图
时间: 2023-07-19 18:43:08 浏览: 179
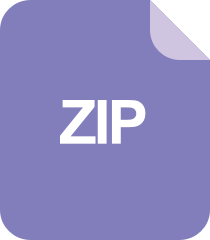
C# 句柄截图(窗口截图)

可以使用C#中的System.Drawing.Bitmap类和Win32 API来获取窗口截图。具体的代码如下:
```csharp
using System;
using System.Drawing;
using System.Runtime.InteropServices;
public class WindowCapture
{
[DllImport("User32.dll")]
public static extern IntPtr GetDC(IntPtr hwnd);
[DllImport("User32.dll")]
public static extern void ReleaseDC(IntPtr hwnd, IntPtr dc);
[DllImport("gdi32.dll")]
public static extern IntPtr CreateCompatibleBitmap(IntPtr dc, int width, int height);
[DllImport("gdi32.dll")]
public static extern IntPtr CreateCompatibleDC(IntPtr dc);
[DllImport("gdi32.dll")]
public static extern IntPtr SelectObject(IntPtr dc, IntPtr obj);
[DllImport("gdi32.dll")]
public static extern bool BitBlt(IntPtr dcDest, int xDest, int yDest, int wDest, int hDest, IntPtr dcSrc, int xSrc, int ySrc, int rasterOp);
public static Bitmap CaptureWindow(IntPtr hwnd)
{
// 获取窗口的设备上下文
IntPtr hdcSrc = GetDC(hwnd);
if (hdcSrc == IntPtr.Zero)
{
return null;
}
// 创建一个兼容的设备上下文和位图
IntPtr hdcDest = CreateCompatibleDC(hdcSrc);
IntPtr hbmDest = CreateCompatibleBitmap(hdcSrc, 100, 100);
if (hdcDest == IntPtr.Zero || hbmDest == IntPtr.Zero)
{
ReleaseDC(hwnd, hdcSrc);
return null;
}
// 将位图选入设备上下文
IntPtr hbmOld = SelectObject(hdcDest, hbmDest);
// 复制窗口的内容到位图中
if (!BitBlt(hdcDest, 0, 0, 100, 100, hdcSrc, 0, 0, 0x00CC0020))
{
SelectObject(hdcDest, hbmOld);
DeleteObject(hbmDest);
DeleteDC(hdcDest);
ReleaseDC(hwnd, hdcSrc);
return null;
}
// 恢复设备上下文并释放资源
SelectObject(hdcDest, hbmOld);
DeleteObject(hbmDest);
DeleteDC(hdcDest);
ReleaseDC(hwnd, hdcSrc);
// 返回位图
return Bitmap.FromHbitmap(hbmDest);
}
}
```
使用方法:
```csharp
IntPtr hwnd = // 窗口句柄
Bitmap bmp = WindowCapture.CaptureWindow(hwnd);
```
其中,hwnd为窗口句柄,bmp为获取到的窗口截图。请注意,此代码仅支持获取窗口的静态截图,无法实时获取窗口的内容。
阅读全文
相关推荐
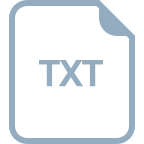
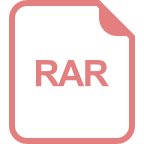
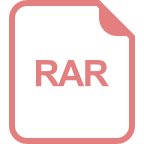






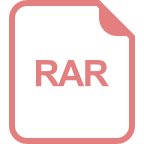



