用java在窗口上编写一个数字时钟
时间: 2023-09-12 11:12:59 浏览: 53
好的,以下是用Java编写数字时钟的示例代码:
```java
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class DigitalClock extends JPanel implements Runnable {
private Thread thread = null;
private SimpleDateFormat dateFormat = new SimpleDateFormat("HH:mm:ss");
private Font clockFont = new Font("Arial", Font.BOLD, 36);
public DigitalClock() {
setBackground(Color.BLACK);
}
public void start() {
if (thread == null) {
thread = new Thread(this);
thread.start();
}
}
public void stop() {
if (thread != null) {
thread = null;
}
}
public void run() {
while (thread != null) {
repaint();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public void paint(Graphics g) {
super.paint(g);
Calendar calendar = Calendar.getInstance();
String time = dateFormat.format(calendar.getTime());
g.setFont(clockFont);
g.setColor(Color.GREEN);
g.drawString(time, 20, 50);
}
public static void main(String[] args) {
JFrame frame = new JFrame("Digital Clock");
DigitalClock digitalClock = new DigitalClock();
frame.add(digitalClock);
frame.setSize(200, 120);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLocationRelativeTo(null);
frame.setVisible(true);
digitalClock.start();
}
}
```
这段代码创建了一个继承自`JPanel`的类`DigitalClock`,并实现了`Runnable`接口。在`run()`方法中,程序不断地重绘数字时钟。在`paint()`方法中,获取当前时间并在窗口上绘制数字时钟。`main()`方法中创建了一个`JFrame`窗口,并将`DigitalClock`添加到窗口中。最后启动`DigitalClock`线程,数字时钟开始运行。
相关推荐
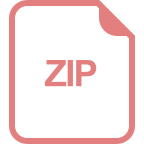
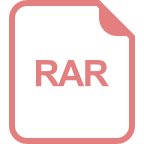














