c# 设计一个能用的任务管理器
时间: 2023-12-14 10:06:10 浏览: 29
以下是一个简单的 C# 任务管理器的设计思路:
1. 创建一个 Task 类,其中包含任务名称、开始时间、结束时间、任务状态等属性。
2. 创建一个 TaskManager 类,其中包含一个 List<Task> tasks 属性,用于存储任务列表。
3. 在 TaskManager 类中,实现添加任务、删除任务、修改任务状态等操作的方法。
4. 创建一个主界面,用于显示任务列表和操作按钮。可以使用 DataGridView 控件来显示任务列表,并添加按钮来触发操作。
5. 当添加、删除、修改任务状态等操作时,需要更新 tasks 列表,并重新在 DataGridView 中显示。
6. 为了保证数据的持久性,可以将 tasks 列表保存到本地文件中,如 XML 文件或 SQLite 数据库。
下面是一个简单的代码示例,实现了添加、删除、修改任务状态等功能:
```csharp
using System;
using System.Collections.Generic;
using System.Windows.Forms;
public class Task
{
public string Name { get; set; }
public DateTime StartTime { get; set; }
public DateTime EndTime { get; set; }
public bool IsCompleted { get; set; }
}
public class TaskManager
{
private List<Task> tasks = new List<Task>();
public void AddTask(Task task)
{
tasks.Add(task);
}
public void RemoveTask(Task task)
{
tasks.Remove(task);
}
public void MarkTaskAsCompleted(Task task)
{
task.IsCompleted = true;
}
public List<Task> GetAllTasks()
{
return tasks;
}
}
public class MainForm : Form
{
private DataGridView dataGridView;
private Button addButton;
private Button removeButton;
private Button completeButton;
private TaskManager taskManager = new TaskManager();
public MainForm()
{
InitializeComponent();
}
private void InitializeComponent()
{
// 初始化控件
dataGridView = new DataGridView();
addButton = new Button();
removeButton = new Button();
completeButton = new Button();
// 设置控件位置和大小
dataGridView.Location = new Point(10, 10);
dataGridView.Size = new Size(400, 200);
addButton.Location = new Point(10, 220);
addButton.Size = new Size(80, 30);
addButton.Text = "添加任务";
addButton.Click += AddButton_Click;
removeButton.Location = new Point(100, 220);
removeButton.Size = new Size(80, 30);
removeButton.Text = "删除任务";
removeButton.Click += RemoveButton_Click;
completeButton.Location = new Point(190, 220);
completeButton.Size = new Size(80, 30);
completeButton.Text = "完成任务";
completeButton.Click += CompleteButton_Click;
// 添加控件到窗口
Controls.Add(dataGridView);
Controls.Add(addButton);
Controls.Add(removeButton);
Controls.Add(completeButton);
// 加载任务列表
LoadTasks();
}
private void LoadTasks()
{
// 从文件或数据库中加载任务列表
List<Task> tasks = LoadTasksFromFile();
// 将任务列表显示在 DataGridView 中
dataGridView.DataSource = tasks;
}
private void AddButton_Click(object sender, EventArgs e)
{
// 弹出对话框,输入任务信息
TaskForm taskForm = new TaskForm();
if (taskForm.ShowDialog() == DialogResult.OK)
{
// 添加任务到任务管理器
Task task = taskForm.GetTask();
taskManager.AddTask(task);
// 更新 DataGridView
dataGridView.DataSource = taskManager.GetAllTasks();
}
}
private void RemoveButton_Click(object sender, EventArgs e)
{
// 获取选中的任务
Task task = (Task)dataGridView.SelectedRows[0].DataBoundItem;
// 从任务管理器中删除任务
taskManager.RemoveTask(task);
// 更新 DataGridView
dataGridView.DataSource = taskManager.GetAllTasks();
}
private void CompleteButton_Click(object sender, EventArgs e)
{
// 获取选中的任务
Task task = (Task)dataGridView.SelectedRows[0].DataBoundItem;
// 标记任务为已完成
taskManager.MarkTaskAsCompleted(task);
// 更新 DataGridView
dataGridView.DataSource = taskManager.GetAllTasks();
}
private List<Task> LoadTasksFromFile()
{
// 从文件或数据库中加载任务列表
// TODO: 实现从文件或数据库中加载任务列表的逻辑
return new List<Task>();
}
}
public class TaskForm : Form
{
private TextBox nameTextBox;
private DateTimePicker startTimePicker;
private DateTimePicker endTimePicker;
private Button okButton;
private Button cancelButton;
public TaskForm()
{
InitializeComponent();
}
private void InitializeComponent()
{
// 初始化控件
nameTextBox = new TextBox();
startTimePicker = new DateTimePicker();
endTimePicker = new DateTimePicker();
okButton = new Button();
cancelButton = new Button();
// 设置控件位置和大小
nameTextBox.Location = new Point(10, 10);
nameTextBox.Size = new Size(200, 30);
startTimePicker.Location = new Point(10, 50);
startTimePicker.Size = new Size(200, 30);
endTimePicker.Location = new Point(10, 90);
endTimePicker.Size = new Size(200, 30);
okButton.Location = new Point(10, 130);
okButton.Size = new Size(80, 30);
okButton.Text = "确定";
okButton.DialogResult = DialogResult.OK;
cancelButton.Location = new Point(100, 130);
cancelButton.Size = new Size(80, 30);
cancelButton.Text = "取消";
cancelButton.DialogResult = DialogResult.Cancel;
// 添加控件到窗口
Controls.Add(nameTextBox);
Controls.Add(startTimePicker);
Controls.Add(endTimePicker);
Controls.Add(okButton);
Controls.Add(cancelButton);
}
public Task GetTask()
{
// 返回输入的任务信息
Task task = new Task();
task.Name = nameTextBox.Text;
task.StartTime = startTimePicker.Value;
task.EndTime = endTimePicker.Value;
task.IsCompleted = false;
return task;
}
}
```
相关推荐
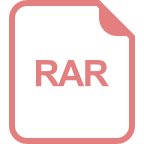
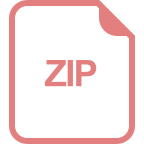














