在pcl中使用,读取点云pcd文件,使用流形最小二乘法进行表面拟合
时间: 2023-12-10 18:41:46 浏览: 107
在PCL中进行表面拟合的方法有很多种,其中流形最小二乘法(Manifold Least Squares)是一种常用的方法。下面是一个读取点云PCD文件并使用流形最小二乘法进行表面拟合的示例代码。
```cpp
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
#include <pcl/surface/mls.h>
int main (int argc, char** argv)
{
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud (new pcl::PointCloud<pcl::PointXYZ>);
// 读取点云文件
if (pcl::io::loadPCDFile<pcl::PointXYZ> ("cloud.pcd", *cloud) == -1) //* load the file
{
PCL_ERROR ("Couldn't read file cloud.pcd \n");
return (-1);
}
// 创建流形最小二乘法对象
pcl::MovingLeastSquares<pcl::PointXYZ, pcl::PointXYZ> mls;
mls.setInputCloud (cloud);
mls.setSearchRadius (0.03); // 设置搜索半径
mls.setPolynomialFit (true); // 使用多项式拟合
mls.setPolynomialOrder (2); // 多项式阶数
mls.setUpsamplingMethod (pcl::MovingLeastSquares<pcl::PointXYZ, pcl::PointXYZ>::SAMPLE_LOCAL_PLANE); // 设置上采样方法
mls.setUpsamplingRadius (0.03); // 上采样搜索半径
mls.setUpsamplingStepSize (0.02); // 上采样步长
// 执行流形最小二乘法
pcl::PointCloud<pcl::PointXYZ>::Ptr mls_points (new pcl::PointCloud<pcl::PointXYZ> ());
mls.process (*mls_points);
// 输出点云
pcl::io::savePCDFileASCII ("mls_points.pcd", *mls_points);
return (0);
}
```
在上面的代码中,我们首先创建了一个`pcl::PointCloud<pcl::PointXYZ>`类型的对象`cloud`,并使用`pcl::io::loadPCDFile`函数从`cloud.pcd`文件中读取点云数据。接着,我们创建了一个`pcl::MovingLeastSquares`类型的对象`mls`,并将点云数据设置为输入。然后,我们设置了一些参数,比如搜索半径、多项式拟合阶数、上采样方法等。最后,我们使用`mls.process`函数进行流形最小二乘法,并将结果保存到`mls_points.pcd`文件中。
需要注意的是,流形最小二乘法是一种计算密集型的算法,处理大规模点云时可能会很慢。因此,在实际应用中,我们需要根据具体情况选择合适的算法和参数。
阅读全文
相关推荐
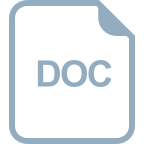
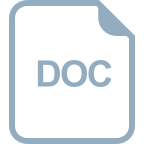
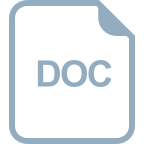
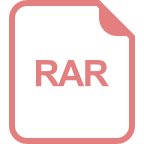



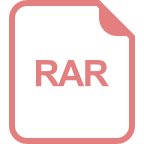
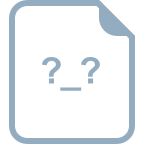
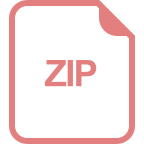








