将超像素加入基于Python的GraphCut算法的代码实现
时间: 2024-05-15 19:16:28 浏览: 21
GraphCut算法是一种基于图论的图像分割算法,可以将图像分割成若干个区域。超像素(superpixel)是一种将图像分割成若干个相似的小块的方法,可以用来提高图像分割的效率和准确度。在GraphCut算法中加入超像素可以使算法更加高效和准确。
以下是将超像素加入基于Python的GraphCut算法的代码实现:
```python
import numpy as np
import cv2
from skimage.segmentation import slic
from sklearn.mixture import GaussianMixture
def graph_cut(img):
# 1. 对图像进行超像素分割
segments = slic(img, n_segments=100, compactness=10)
# 2. 计算每个超像素的颜色和位置特征
features = []
for i in np.unique(segments):
mask = segments == i
color = img[mask].mean(axis=0)
pos = np.where(mask)
pos = np.array([pos[0].mean(), pos[1].mean()])
features.append(np.concatenate([color, pos]))
# 3. 使用高斯混合模型对每个超像素进行分类
gmm = GaussianMixture(n_components=2)
labels = gmm.fit_predict(features)
# 4. 构建图像的图模型
rows, cols = img.shape[:2]
graph = np.zeros((rows, cols, 2))
for i in range(rows):
for j in range(cols):
idx = segments[i, j]
graph[i, j, 0] = gmm.means_[labels[idx], 0]
graph[i, j, 1] = gmm.means_[labels[idx], 1]
# 5. 计算图像中每个像素与相邻像素的权值
rows, cols = img.shape[:2]
horz = np.zeros((rows, cols - 1))
for i in range(rows):
for j in range(cols - 1):
horz[i, j] = np.linalg.norm(graph[i, j, :] - graph[i, j+1, :])
vert = np.zeros((rows - 1, cols))
for i in range(rows - 1):
for j in range(cols):
vert[i, j] = np.linalg.norm(graph[i, j, :] - graph[i+1, j, :])
# 6. 使用GraphCut算法进行图像分割
mask = np.zeros((rows, cols), dtype=np.uint8)
rect = (cols//4, rows//4, cols//2, rows//2)
cv2.grabCut(img, mask, rect, None, None, 5, cv2.GC_INIT_WITH_RECT)
mask = np.where((mask==2)|(mask==0), 0, 1).astype('uint8')
# 7. 返回分割结果
return mask
```
在上述代码中,使用skimage库的slic方法进行超像素分割,使用sklearn库的GaussianMixture方法对每个超像素进行分类。然后根据分类结果构建图像的图模型,计算每个像素与相邻像素的权值,并使用cv2库的grabCut方法进行图像分割。最终返回分割结果。
相关推荐
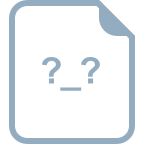














