java 逐行读取.log文件
时间: 2023-07-12 08:32:30 浏览: 309
可以使用Java的BufferedReader类来逐行读取.log文件。以下是一个示例代码:
```
import java.io.*;
public class ReadLog {
public static void main(String[] args) {
try {
BufferedReader br = new BufferedReader(new FileReader("file.log"));
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
br.close();
} catch (IOException e) {
System.out.println("Error reading file: " + e.getMessage());
}
}
}
```
这个代码会打开名为file.log的文件,并逐行读取其中的内容。每读取一行,就会将其输出到控制台。在处理完文件后,代码会关闭BufferedReader对象。
相关问题
Java 读取超大log4j 怎么提高速度
要提高Java读取超大log4j日志文件的速度,可以考虑以下几个方面的优化:
1. 使用BufferedReader:使用BufferedReader替代普通的FileReader,这样可以减少I/O操作次数,提高读取效率。例如:
```java
BufferedReader reader = new BufferedReader(new FileReader("path/to/logfile.log"));
String line;
while ((line = reader.readLine()) != null) {
// 处理日志行
}
reader.close();
```
2. 逐行读取:对于超大日志文件,不要一次性读取整个文件,而是逐行读取。这样可以降低内存的压力,提高处理速度。
3. 使用多线程:如果处理日志的逻辑可以并行执行,可以考虑使用多线程来加速处理速度。例如,可以将日志文件按照一定的规则分割成多个部分,然后使用多个线程并行读取和处理。
4. 使用正则表达式匹配:如果你只需要读取符合某种特定模式的日志行,可以使用正则表达式来进行匹配,这样可以过滤掉不符合条件的日志行,提高读取速度。
5. 增加内存限制:通过调整JVM的内存限制参数,如-Xmx和-Xms等,可以为Java程序提供更大的堆空间,减少频繁的垃圾回收操作,提高读取速度。
6. 使用NIO:使用Java的NIO(New I/O)库,通过Channel和Buffer的方式进行文件读取,可以提高文件读取效率。例如使用FileChannel和ByteBuffer来读取日志文件。
以上是一些常见的优化策略,具体的优化方案需要根据实际情况进行调整和测试,以达到最佳的读取速度。
在hadoop中mapreduce,如何统计一个文件email_log.txt文件内容中@字符的出现次数,并用键值对表示出来
在Hadoop MapReduce框架下,要统计一个文本文件中`@`字符的出现次数,你可以按照以下步骤设计Map和Reduce阶段:
1. **Mapper阶段**:
- 使用`TextInputFormat`读取文件,例如:`FileInputFormat.addInputPath(job, new Path("email_log.txt"))`
- 创建一个自定义的Mapper类,`Mapper extends Mapper<Object, Text, Key, Value>`,这里Key可以是IntWritable(0),Value可以是Text。
- 在`map()`函数里,逐行读取输入的文本,对于每行,分割成单词并检查是否包含`@`。如果是,就创建一个新的键值对`(1, word)`(假设我们想要计算每个`@`计数),如果不是,则忽略。
```java
private static class CountAtSymbolMapper extends Mapper<Object, Text, IntWritable, Text> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
@Override
protected void map(Object key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
for (String word : line.split("\\s+")) {
if (word.contains("@")) {
context.write(one, new Text(word));
}
}
}
}
```
2. **Reducer阶段**:
- 创建一个Reducer类,`Reducer<IntWritable, Text, IntWritable, Text>`, 用于累加每个`@`出现的次数。
- 在`reduce()`函数里,接收来自Mapper的所有`@`计数,将它们相加并作为新的键值对`(count, "@")`写入到结果。
```java
private static class SumReducer extends Reducer<IntWritable, Text, IntWritable, Text> {
private IntWritable result = new IntWritable();
@Override
protected void reduce(IntWritable key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
int count = 0;
for (Text value : values) {
count += value.toString().equals("@") ? 1 : 0; // 如果value等于"@",计数加一
}
result.set(count);
context.write(key, new Text(result.toString()));
}
}
```
3. **Job配置**:
- 设置Mapper和Reducer类,以及适当的OutputFormat,如`TextOutputFormat`。
- 调用`job.setOutputKeyClass(IntWritable.class)` 和 `job.setOutputValueClass(Text.class)`设置输出键值对的类型。
- 运行任务:`job.waitForCompletion(true)`。
运行完成后,`key`字段将是总的`@`次数,`value`字段总是相同的字符串`"@"`。
阅读全文
相关推荐
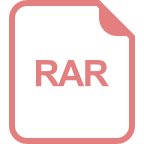
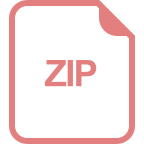
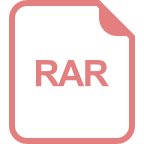
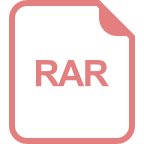
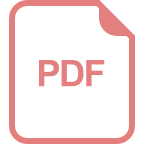

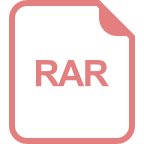
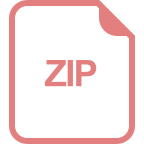
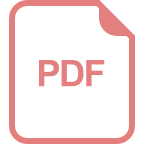
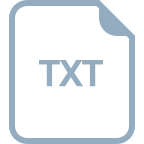
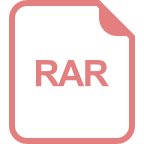
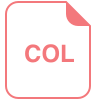
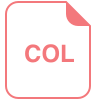
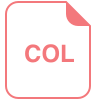
