深度遍历和广度遍历实现二叉树深度的计算
时间: 2023-07-24 08:08:04 浏览: 137
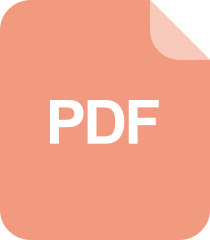
Java实现二叉树的深度优先遍历和广度优先遍历算法示例

深度遍历和广度遍历都可以用来实现二叉树深度的计算。
深度遍历主要有前序遍历、中序遍历和后序遍历。其中,前序遍历的顺序是:根节点 -> 左子树 -> 右子树;中序遍历的顺序是:左子树 -> 根节点 -> 右子树;后序遍历的顺序是:左子树 -> 右子树 -> 根节点。通过这三种遍历方式,我们可以得到二叉树的深度。
具体实现方法如下:
1. 前序遍历实现深度计算:
```python
def preorder_depth(root):
if not root:
return 0
left_depth = preorder_depth(root.left)
right_depth = preorder_depth(root.right)
return max(left_depth, right_depth) + 1
```
2. 广度遍历实现深度计算:
```python
def bfs_depth(root):
if not root:
return 0
queue = [(root, 1)]
depth = 0
while queue:
node, level = queue.pop(0)
if node.left:
queue.append((node.left, level+1))
if node.right:
queue.append((node.right, level+1))
depth = level
return depth
```
以上两种方式都可以实现二叉树深度的计算,其中前序遍历是深度优先遍历,广度遍历是广度优先遍历。
阅读全文
相关推荐
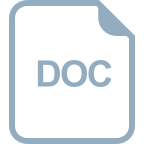
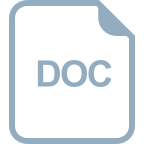

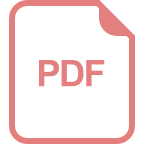
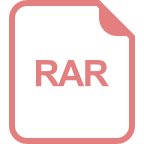


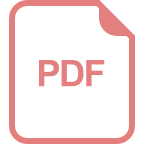
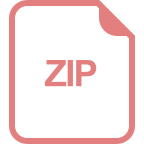
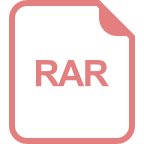
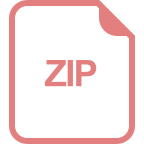
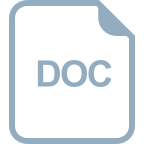
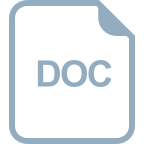



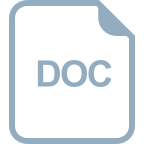
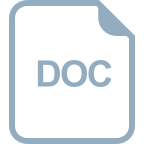